Class Property in Python
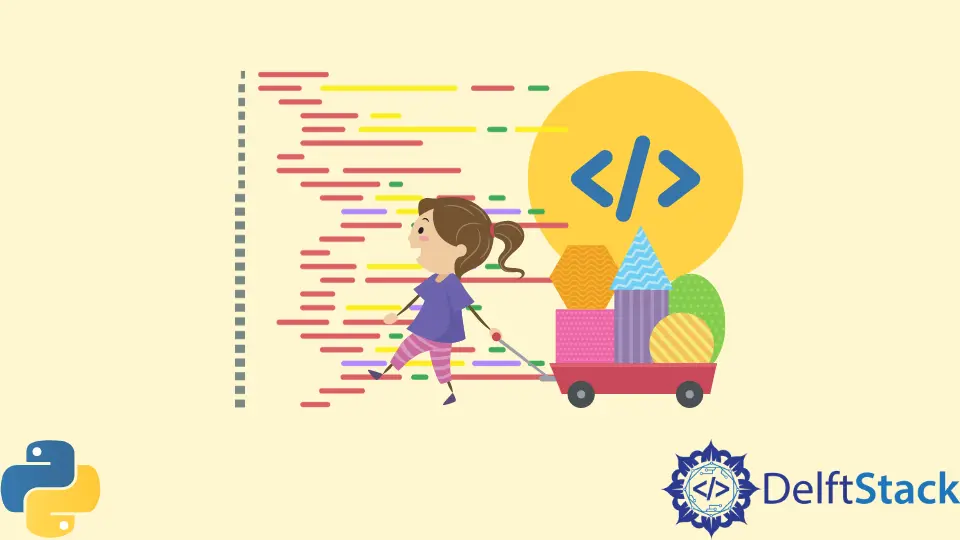
Python is an object-oriented programming language that has almost everything based on an object with properties and methods. A class is like an object manufacturer or a blueprint for creating objects. The property in a class is an element or feature that characterize classes. Furthermore, in Python, classes are sets of various objects, and an instance of a class is a particular object which directly belongs to only one class.
This article will introduce the Python property
decorator. It is easy to use the properties in Python using setters
and getters
methods in object-oriented programming. Python programming language uses a built-in property
decorator that uses the getter and setters methods, making the flow of code much easier in Object-Oriented Programming. You initiate a property by calling the property()
built-in function, passing in three methods: getter
, setter
, and deleter
. It is considered to be more advanced and efficient than the procedural style of programming.
A property is a class member that is intermediate between a field and a method. The property()
function uses the setter, getter, and deleter function.
Class Property in Python
We have created the Animal
class in this code block, and we have added one setter
and getter
for this class. In the setter
function, we are setting the name of the Animal
, and in getter
we are getting the name that we have set from the getter method. Then we have created the Animal object. In this object, we have passed the animal name as Dog. Furthermore, the animal’s name has been printed from the function using the value() getter function. Also, in animal class, the constructor method that sets the value according to the class object passed, write the @property
decorator to make a class property.
class Animal:
def __init__(self, Name):
self.Name = Name
@property
def value(self):
return self.Name
animalObject = Animal("Dog")
print(animalObject.value)
Output:
Dog
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn