How to Check Variable Is String or Not in Python
-
Use the
type()
Function to Check if a Variable Is a String or Not -
Use the
isinstance()
Function to Check if a Variable Is a String or Not
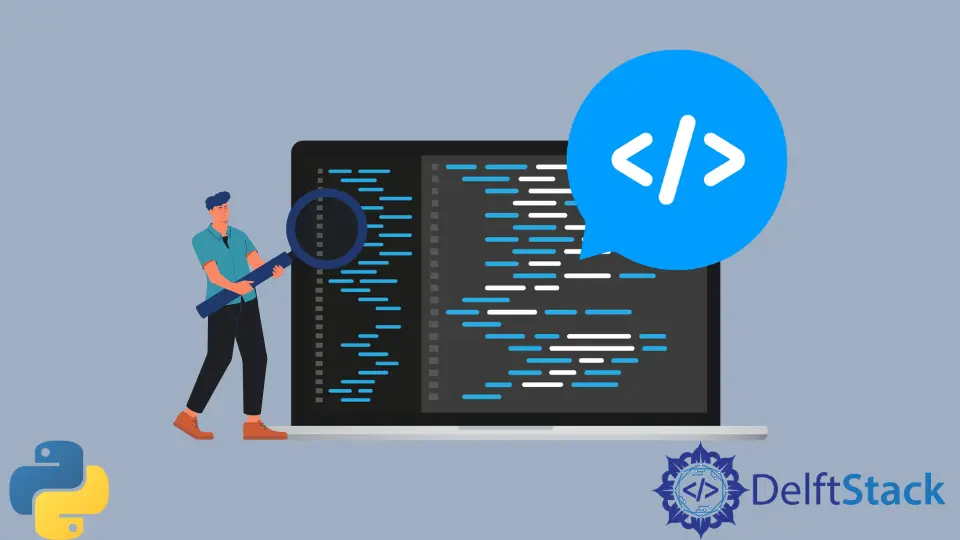
The string datatype is used to represent a collection of characters. This tutorial will discuss how to check if a variable is a string type or not.
Use the type()
Function to Check if a Variable Is a String or Not
The type()
function returns the class type of passed variable. The following code shows how to use this function to check if a variable is a string or not.
value = "Yes String"
if type(value) == str:
print("True")
else:
print("False")
Output:
True
However, it is worth noting that this method is generally discouraged and is termed unidiomatic in Python. The reason behind that is because the ==
operator compares the variable for only the string class and will return False
for all its subclasses.
Use the isinstance()
Function to Check if a Variable Is a String or Not
It is therefore encouraged to use the isinstance()
function over the traditional type()
. The isinstance()
function checks whether an object belongs to the specified subclass. The following code snippet will explain how we can use it to check for string objects.
value = "Yes String"
if isinstance(value, str):
print("True")
else:
print("False")
Output:
True
In Python 2, we can use the basestring
class, which is an abstract class for str
and unicode
, to test whether an object is an instance of str
or unicode
. For example,
value = "Yes String"
if isinstance(value, basestring):
print("True")
else:
print("False")
Output:
True
For using the above method in Python 3, we can use the six
module. This module has functions that allow us to write code compatible with both Python 2 and 3.
The string_types()
function returns all the possible types for string data. For example,
import six
value = "Yes String"
if isinstance(value, six.string_types):
print("True")
else:
print("False")
Output:
True
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn