How to Check List Is Empty in Python
-
Method 1: Using the
if
Statement -
Method 2: Using the
len()
Function -
Method 3: Using the
list
Constructor -
Method 4: Using the
any()
Function - Conclusion
- FAQ
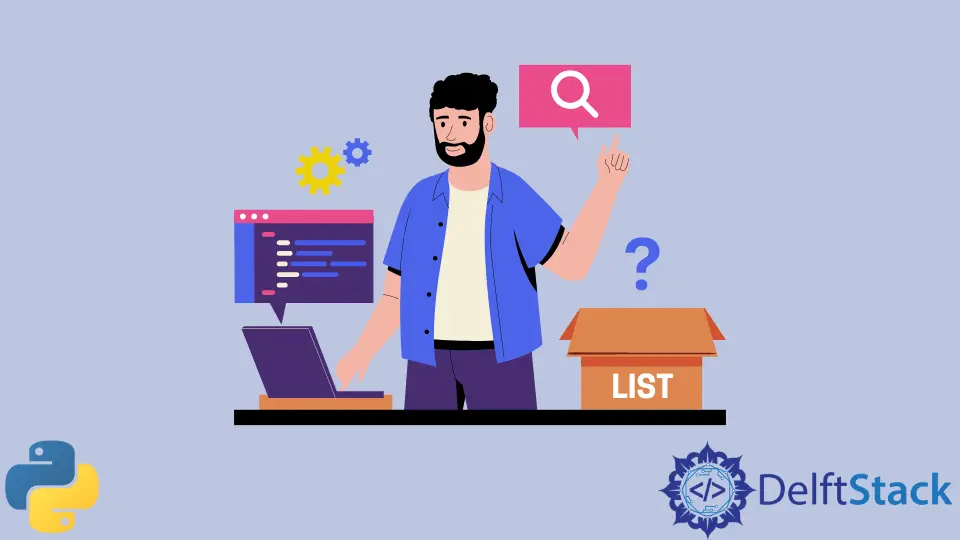
Checking if a list is empty in Python is a fundamental skill that every programmer should master. Whether you’re a beginner learning the ropes or an experienced developer, understanding how to assess the contents of a list can save you time and prevent errors in your code.
In this tutorial, we’ll explore various methods to determine if a list is empty. We’ll provide clear examples and explanations to help you grasp these concepts easily. By the end of this article, you’ll be equipped with the knowledge to confidently check for empty lists in your Python programs.
Method 1: Using the if
Statement
One of the most straightforward ways to check if a list is empty is by utilizing an if
statement. In Python, an empty list evaluates to False
, while a non-empty list evaluates to True
. This means you can directly use the list in a condition.
my_list = []
if not my_list:
print("The list is empty.")
else:
print("The list is not empty.")
Output:
The list is empty.
In this example, we create an empty list called my_list
. The if not my_list:
statement checks if the list is empty. Since my_list
is indeed empty, the code inside the if
block executes, printing “The list is empty.” If the list contained any elements, the else block would run, indicating that the list is not empty. This method is not only concise but also very readable, making it a preferred choice for many developers.
Method 2: Using the len()
Function
Another effective way to check if a list is empty is by using the len()
function. This built-in function returns the number of items in a list. If the length is zero, the list is empty.
my_list = []
if len(my_list) == 0:
print("The list is empty.")
else:
print("The list is not empty.")
Output:
The list is empty.
In this example, we again initialize an empty list, my_list
. We then use the len()
function to check the number of elements in the list. If len(my_list)
equals zero, we print that the list is empty. Otherwise, we indicate that the list contains items. This method is clear and explicit, making it easy for anyone reading your code to understand the intent.
Method 3: Using the list
Constructor
You can also check if a list is empty by creating a new list using the list()
constructor. If the list is empty, the constructor will return an empty list, which you can compare to the original list.
my_list = []
if my_list == list():
print("The list is empty.")
else:
print("The list is not empty.")
Output:
The list is empty.
In this case, we compare my_list
to a new empty list created by list()
. If they are equal, it means my_list
is empty. This method might be less common but can still be useful in certain scenarios where you prefer explicit comparisons. It’s worth noting that while this approach works, it’s often more efficient and clearer to use the previous methods.
Method 4: Using the any()
Function
The any()
function can also be utilized to check if a list is empty. This function returns True
if at least one element in the iterable is true. For an empty list, it will return False
.
my_list = []
if not any(my_list):
print("The list is empty.")
else:
print("The list is not empty.")
Output:
The list is empty.
In this example, we use any(my_list)
to check if there are any truthy values in my_list
. Since the list is empty, any(my_list)
returns False
, leading to the conclusion that the list is indeed empty. This method is particularly handy when you want to check for truthy values in a list, but it can also serve as a quick way to check for emptiness.
Conclusion
In summary, checking if a list is empty in Python is a crucial skill for any programmer. Whether you opt for the straightforward if
statement, the len()
function, the list()
constructor, or the any()
function, each method has its merits. By understanding these techniques, you can write cleaner, more efficient code that handles lists effectively. With practice, you’ll find the method that best suits your coding style and needs. Happy coding!
FAQ
-
How do I check if a list contains specific elements?
You can use thein
keyword to check if specific elements are in a list. -
Can I check if a list is empty without using an if statement?
Yes, you can directly use the list in a boolean context, as an empty list evaluates toFalse
. -
What happens if I try to access an index in an empty list?
Attempting to access an index in an empty list will raise anIndexError
. -
Are there performance differences between these methods?
Generally, theif not my_list
method is the most efficient and preferred for checking list emptiness. -
Can I use these methods for other data types, like tuples or strings?
Yes, similar techniques can be applied to other data types, as they also have truthy and falsy evaluations.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python