Ceiling Division in Python
-
Ceiling Division Using the
//
Operator in Python -
Ceiling Division Using the
math.ceil()
Function in Python
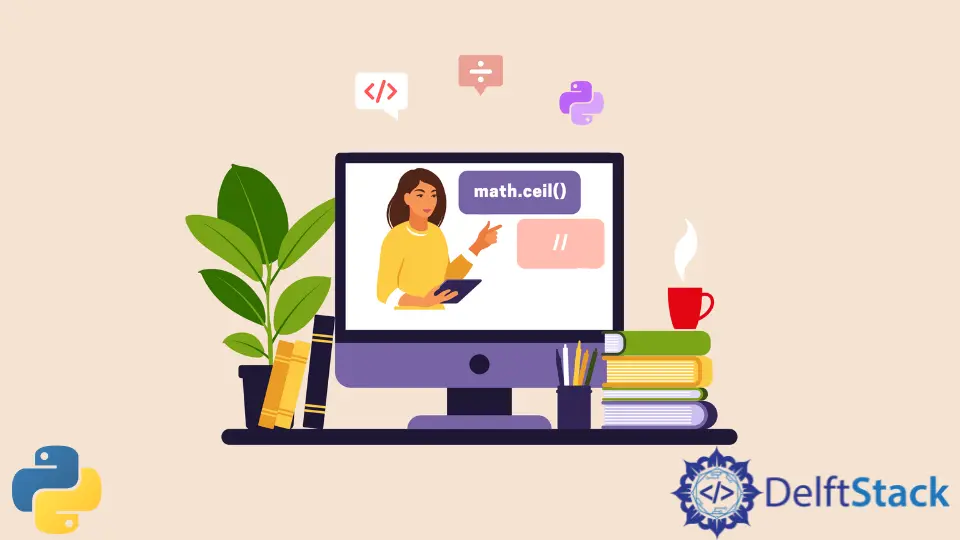
Ceiling division returns the closest integer greater than or equal to the current answer or quotient. In Python, we have an operator //
for floor division, but no such operator exists for the ceiling division. This article will talk about different ways in which we can perform ceiling division in Python.
Ceiling Division Using the //
Operator in Python
We can use so math and floor division //
to perform ceiling division in Python. Refer to the following code.
def ceil(a, b):
return -1 * (-a // b)
print(ceil(1, 2))
print(ceil(5, 4))
print(ceil(7, 2))
print(ceil(5, 3))
print(ceil(121, 10))
Output:
1
2
4
2
13
What we did is as follows -
-a // b
will return the same answer but with the opposite sign as compared to that ofa // b
.- Since on the negative side,
-a
is greater than-(a + 1)
, wherea
is a positive number, the//
operator will return an integer just smaller than the actual answer. For example, if the answer from the normal division was-1.25
, the floor value returned will be-2
(closest smallest integer to-1.25
). - By multiplying
-1
to the intermediate answer or result of(-a // b)
, we will get the answer with its expected sign. The returned value is essentially the result of ceiling division.
Ceiling Division Using the math.ceil()
Function in Python
Python has a math
package that is filled with functions and utilities to perform mathematical operations. One such function is the ceil()
function. This function returns the ceiling value of the passed number. For example, if we pass 2.3
to this function, it will return 3
. We will pass the result of normal division to this function and return its ceil value. Refer to the following code for some more examples and their usage.
from math import ceil
print(ceil(1 / 2))
print(ceil(5 / 4))
print(ceil(7 / 2))
print(ceil(5 / 3))
print(ceil(121 / 10))
Output:
1
2
4
2
13