How to Calculate the Arithmetic Mean in Python
- Use the Mathematical Formula to Calculate the Arithmetic Mean in Python
-
Use the
numpy.mean()
Function to Calculate the Arithmetic Mean in Python -
Use the
statistics.mean()
Function to Calculate the Arithmetic Mean in Python -
Use the
scipy.mean()
Function to Calculate the Arithmetic Mean in Python
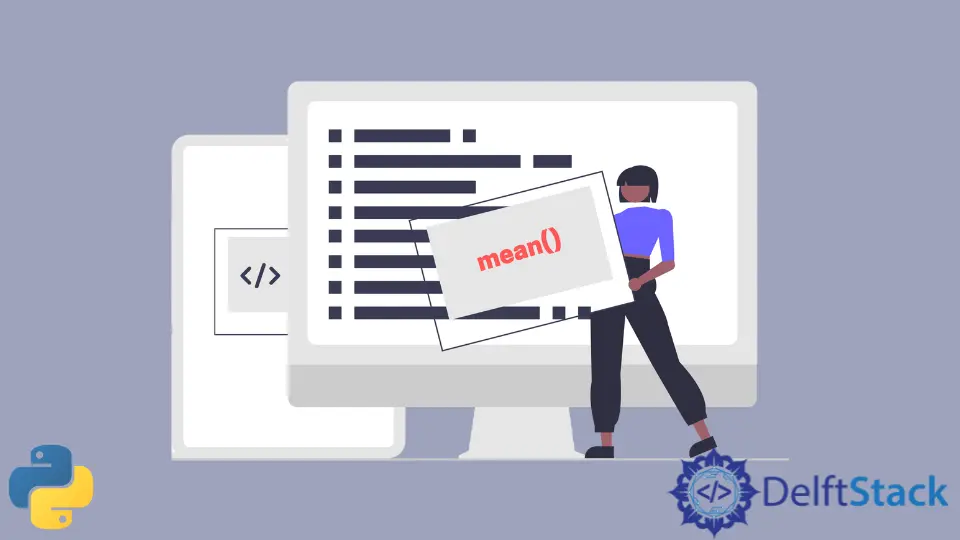
The term arithmetic mean is the average of the numbers. The mathematical formula to determine the Arithmetic Mean is to divide the sum of the numbers by the count. It is determined in Python in the following ways.
- Use the mathematical formula.
- Use the mean() function from Python’s standard libraries like
NumPy
,statistics
,scipy
.
Use the Mathematical Formula to Calculate the Arithmetic Mean in Python
Follow this program to use the mathematical formula.
listnumbers = [1, 2, 4]
print("The mean is =", sum(listnumbers) / len(listnumbers))
Output:
The mean is = 2.3333333333333335
Use the numpy.mean()
Function to Calculate the Arithmetic Mean in Python
The NumPy
standard library contains the mean()
function used to determine the Arithmetic Mean in Python. For this, import the NumPy
library first. See the example below.
import numpy
listnumbers = [1, 2, 4]
print("The mean is =", numpy.mean(listnumbers))
Output:
The mean is = 2.3333333333333335
Use the statistics.mean()
Function to Calculate the Arithmetic Mean in Python
The statistics
library contains the mean()
function used to determine the Arithmetic Mean. For this, import the statistics
library first. Follow the example below.
import statistics
listnumbers = [1, 2, 4]
print("The mean is =", statistics.mean(listnumbers))
Output:
The mean is = 2.3333333333333335
Use the scipy.mean()
Function to Calculate the Arithmetic Mean in Python
The scipy
library contains the mean()
function used to determine the mean. For this, import the scipy
library first. Here’s an example.
import scipy
listnumbers = [1, 2, 4]
print("The mean is =", scipy.mean(listnumbers))
Output:
The mean is = 2.3333333333333335