Built-In Identity Function in Python
- Understanding the Identity Function
- Using Lambda Functions to Create an Identity Function
-
Implementing the Identity Function with the
id()
Function -
Using the
operator
Module for Identity - Conclusion
- FAQ
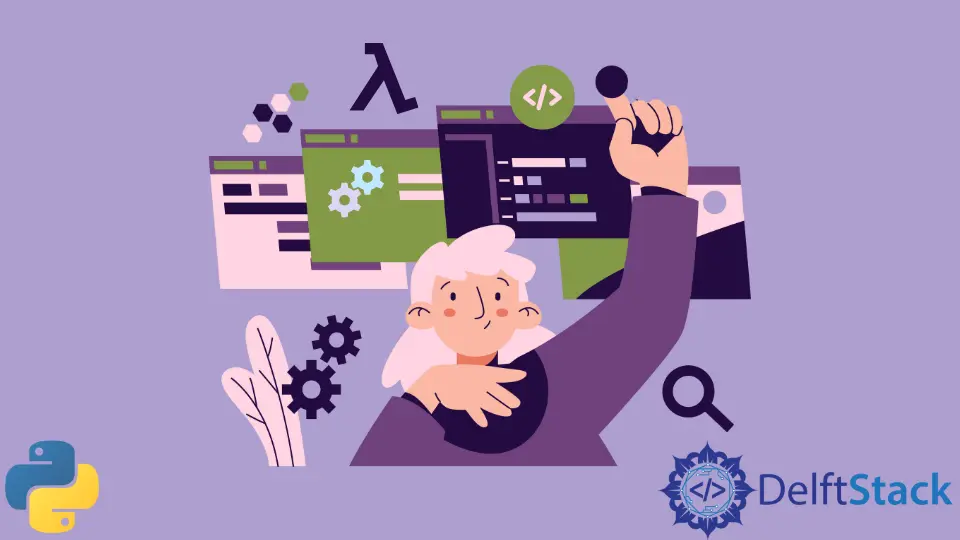
In the world of programming, understanding how functions operate is crucial. One such function that often piques the interest of Python developers is the identity function. But does Python have a built-in identity function?
This article aims to clarify that while exploring various methods to achieve similar outcomes. We will dive into the concept of identity functions, their significance in programming, and how you can leverage them in your Python projects. By the end of this tutorial, you will have a solid grasp of the built-in identity function in Python and how to implement it effectively.
Understanding the Identity Function
An identity function is a function that always returns the same value that was used as its input. In mathematical terms, it can be represented as f(x) = x. In Python, while there isn’t a dedicated built-in function named “identity,” the functionality can be easily achieved using lambda functions or the standard id()
function. The id()
function returns the identity of an object, which is a unique integer for each object during its lifetime.
Let’s explore how you can effectively utilize these concepts in Python.
Using Lambda Functions to Create an Identity Function
One of the simplest ways to create an identity function in Python is by using a lambda function. Lambda functions are anonymous functions defined with the lambda
keyword. They are often used for short, throwaway functions. Here’s how you can create an identity function using a lambda:
identity = lambda x: x
result = identity(10)
print(result)
Output:
10
In this code, we define a lambda function named identity
that takes one argument, x
, and returns it unchanged. When we call identity(10)
, it returns the value 10. This is a straightforward implementation of an identity function, showcasing how you can create simple and effective functions in Python.
Lambda functions are particularly useful when you need a quick function for operations like sorting or filtering data. They can be passed as arguments to higher-order functions, making them versatile for various programming tasks. While they may not be as readable as named functions, their brevity can lead to cleaner code in some scenarios.
Implementing the Identity Function with the id()
Function
Another approach to achieving the identity function’s behavior is by using Python’s built-in id()
function. While id()
does not return the input value directly, it returns a unique identifier for the object, which can serve as a form of identity representation. Here’s how you can use it:
value = "Hello, World!"
identity_id = id(value)
print(identity_id)
Output:
140676879732416
In this example, we define a string variable value
and then call the id()
function to retrieve its unique identifier. The output is an integer that represents the memory address of the object. While this doesn’t return the original value, it does provide a way to identify the object uniquely throughout its lifecycle.
The id()
function can be particularly useful in debugging and understanding object references in Python. It helps you see whether two variables point to the same object or if they are distinct entities. This can be crucial for optimizing memory usage and understanding how Python manages object lifetimes.
Using the operator
Module for Identity
Python also allows you to create an identity function using the operator
module, which includes a variety of functions that correspond to standard operations. The operator
module has an itemgetter
function that can be used to create an identity function. Here’s how:
import operator
identity_func = operator.itemgetter(0)
result = identity_func([42])
print(result)
Output:
42
In this code, we import the operator
module and use itemgetter(0)
to create an identity-like function. When we pass a list containing the number 42, the function returns the first item, which is 42 itself. While this is not a traditional identity function, it demonstrates how you can leverage existing modules to achieve similar functionality.
Using the operator
module can be advantageous when you are working with data structures like lists or dictionaries. It provides a more functional programming approach, allowing you to create reusable components without explicitly defining new functions.
Conclusion
In summary, Python does not have a built-in identity function in the traditional sense, but it offers various ways to achieve similar functionality. You can create an identity function using lambda expressions, utilize the id()
function to get unique identifiers, or leverage the operator
module for a more functional approach. Understanding these methods will enhance your programming skills and allow you to write cleaner, more efficient code.
By mastering the concept of identity functions in Python, you can improve your problem-solving capabilities and gain deeper insights into how Python handles objects and memory. Whether you’re a beginner or an experienced developer, these techniques will serve you well in your coding journey.
FAQ
-
Does Python have a built-in identity function?
No, Python does not have a specific built-in identity function, but similar functionality can be achieved using lambda functions or theid()
function. -
What is the purpose of the
id()
function in Python?
Theid()
function returns a unique identifier for an object, which can be useful for tracking object identities and memory management. -
Can I create an identity function using the
operator
module?
Yes, theoperator
module provides various functions, includingitemgetter
, which can be used to mimic identity function behavior. -
What are lambda functions in Python?
Lambda functions are anonymous functions defined with thelambda
keyword, typically used for short, throwaway functions. -
Why is understanding identity functions important in programming?
Understanding identity functions helps in grasping how objects are managed in memory, making it easier to write efficient and effective code.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn