How to Fix the SyntaxError: 'break' Outside Loop Error in Python
- Loops and Conditional Statements in Python
-
Fix the
SyntaxError: 'break' outside loop
Error in Python
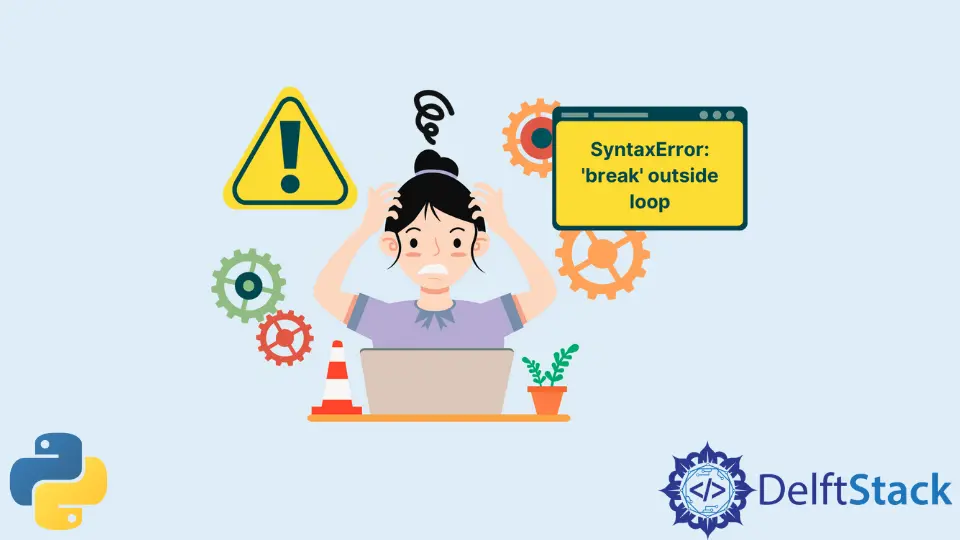
This tutorial will discuss Python’s SyntaxError: 'break' outside loop
error.
Loops and Conditional Statements in Python
Loops and conditional statements are a very integral part of any programming language.
Python provides two loops for
and while
that can execute a set of statements till a condition is met. The if-else
statements are very common for executing some statements based on a condition.
The break
statement is handy when working with loops; it can be used to break out of a loop. This means the control flow is shifted out of the loop whenever the break
statement is encountered and the following statement is executed.
For example,
for i in range(2):
print(i)
break
Output:
0
Fix the SyntaxError: 'break' outside loop
Error in Python
This error is caused due to a violation of the defined syntax of Python. As the error suggests, it occurs because the break
statement is not within the loop but is rather outside the loop.
For example,
a = 7
if a > 5:
break
Output:
SyntaxError: 'break' outside loop
The break
statement can only exist in a loop. In the above example, we put it in the if
statement, so the error was raised.
The fix for this error is simple, use the break
statement only with a loop.
We can put the if
statement within a loop to avoid this error.
See the code below.
a = 7
while True:
if a > 5:
break
print("Break Success")
Output:
Break Success
The above example created a loop where the condition is always true. We used an if
statement to check the condition.
Since the condition is true, the break
statement is executed, and we break out of the loop.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python