How to Break Out of Multiple Loops in Python
-
Break Out of Multiple Loops With the
return
Statement in Python -
Break Out of Multiple Loops With the
break
Keyword in Python
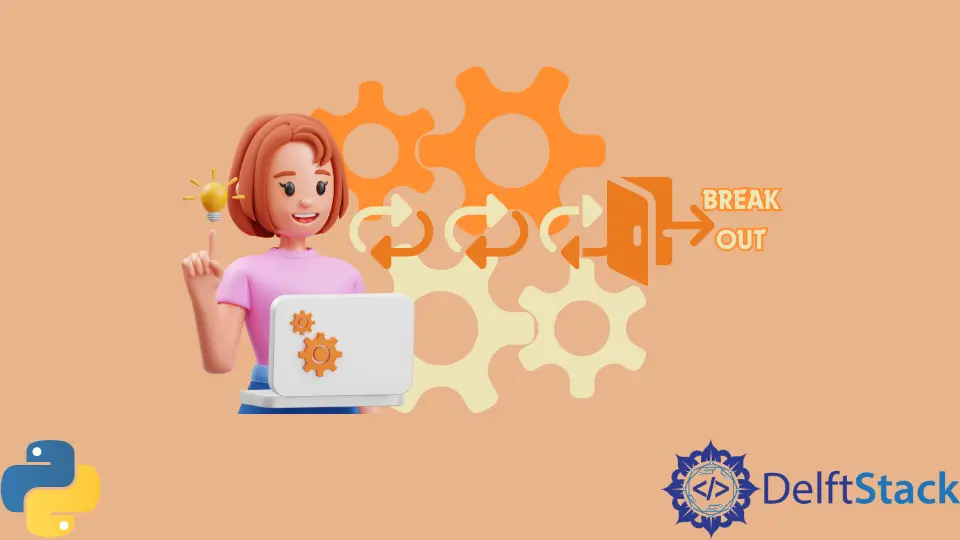
In this tutorial, we will discuss methods to break out of multiple loops in Python.
Break Out of Multiple Loops With the return
Statement in Python
In this method, we can write the nested loop inside a user-defined function and use the return
statement to exit the nested loops. The following code example shows us how we can use the return
statement to break out of Python’s multiple loops.
list1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
def search(n):
for x in range(3):
for y in range(3):
if list1[x][y] == n:
return "Found"
return "Not Found"
result = search(10)
print(result)
Output:
Found
In the above code, we first initialize a 2D list and define a function search(n)
that uses a nested loop to search for a specific value inside the list1
. The return
statement is used to exit the nested loop. The function search(n)
returns Found
if the value is found in the list and returns Not Found
if the value is not found in the list.
Break Out of Multiple Loops With the break
Keyword in Python
We can also use the for/else
loop for exiting a nested loop. The else
clause executes after the successful completion of the for
. If the for
loop is broken, the else
is not executed. The following code example shows us how we can use the for/else
loop to break out multiple loops in Python.
list1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
n = 6
for x in range(3):
for y in range(3):
if list1[x][y] == n:
print("Found")
break
else:
continue
break
Output:
Found
In the above code, we first initialize a 2D list and run a nested loop to search for a specific value in list1
. The outer loop is just a simple for
loop. The inner for
loop has an else
clause with it. The code breaks out of the nested loop if the value is found, and keeps on going until completion if the value is not found.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn