Binary Numbers Representation in Python
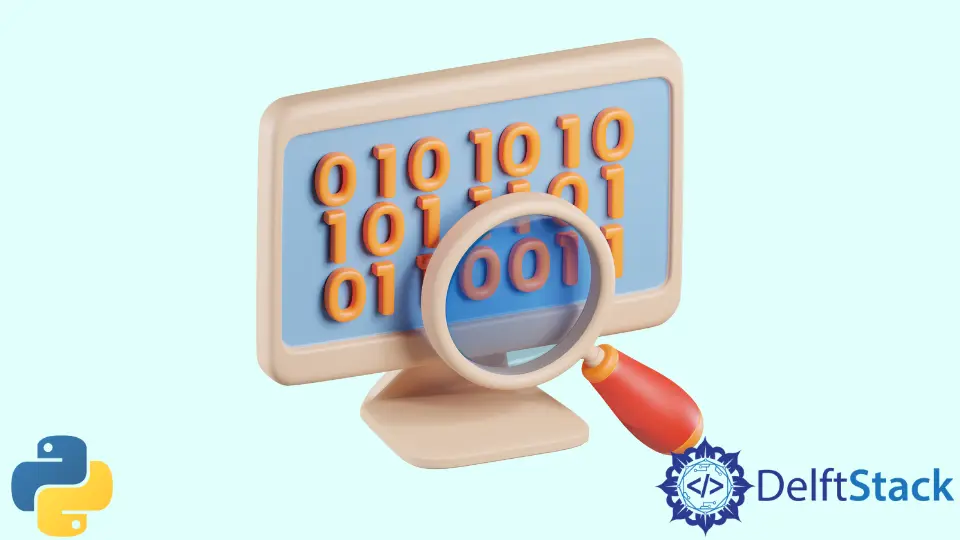
Binary
digits consist of only two values, 0 and 1. From these two values, we can create any combinations of values that exist in the world.
To work with the binary
numbers, we can have the naive approach and the Python built-in functions libraries. The Naive approach begins from the last digits of the binary numbers and follows the carry forward method to other digits of the number whereas, the Python built-in-in functions are used to convert the binary
numbers to decimal and then perform the desired actions.
Python bitwise
operators help you to work with binary numbers in the most efficient way. These binary numbers work the same as decimal numbers, and the only difference with the decimal number is the data representation. So, in this article, we will see how to manipulate the bitwise
operators in Python.
Sum of Two Binary
Numbers in Python
This method will first initialize the two binary
numbers as value1
and value2
. Furthermore, by using the built-in Python functions, we will calculate the sum of the binary numbers.
Example Codes:
# python 3.x
value1 = "0b100"
value2 = "0b110"
sumOfBinaryNumber = bin(int(value1, 2) + int(value2, 2))
print(sumOfBinaryNumber[2:])
Output:
1010
Bitwise Representation in Python
In this technique, we will first initialize the two binary numbers as value1 and value 2. Furthermore, by using the bitwise
operations, we will manipulate these numbers as per requirements. In the below code, ~
is used for NOT operation, ^
for XOR operation, |
for OR operation, and &
for AND operation.
Example Codes:
# python 3.x
value1 = int("1101", 2)
value2 = int("00100110", 2)
print("value1 & value2 =", bin(value1 & value2))
print("value1 | value2 =", bin(value1 | value2))
print("value1 ^ value2 =", bin(value1 ^ value2))
print("~value1 ^ value2 =", bin(~value1 ^ value2))
print("~value1 =", bin(~value1))
print("~value2 =", bin(~value2))
Output:
a & b = 0b100
a | b = 0b101111
a ^ b = 0b101011
~a ^ b = -0b101100
~a = -0b1110
~b = -0b100111
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn