How to Fix AttributeError: 'NoneType' Object Has No Attribute 'Text' in Python
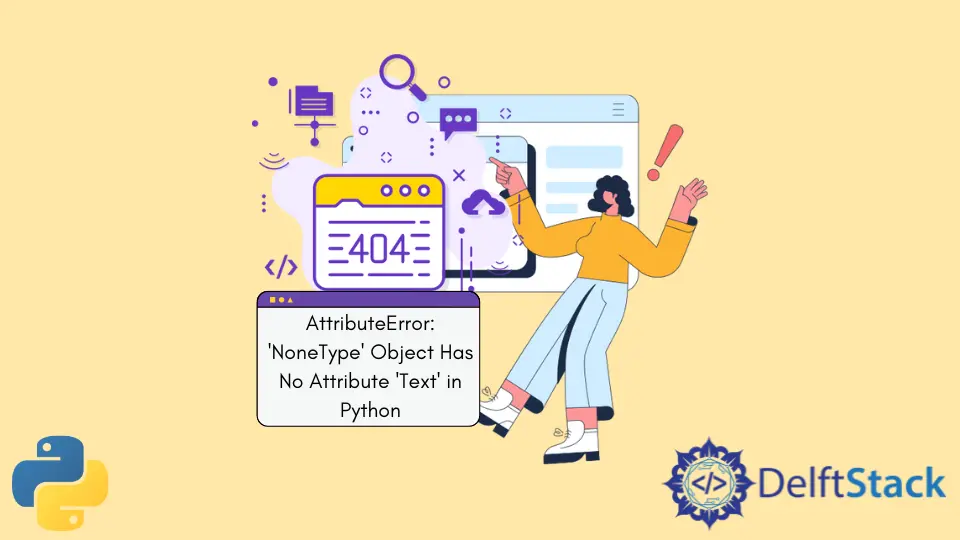
This error happens when you try to call a method from an object that is None or not initiated. Before calling a method, you need to check if the object is None
or not to eliminate this error; after that, call the desired method.
AttributeError
is an exception you get while you call the attribute, but it is not supported or present in the class definition.
Causes and Solutions of the AttributeError: 'NoneType' object has no attribute 'text'
in Python
This error is common while you’re doing web scraping or XML parsing. During the parsing, you’ll get this error if you get unstructured data.
Here are some more reasons:
- Data that JavaScript dynamically rendered.
- Scraping multiple pages with the same data.
- While parsing XML, if the node you are searching is not present.
Here are the common solutions you can try to get rid of the error:
- Check if the element exists before calling any attribute of it.
- Check the response to the request.
an Example Code
As this error often comes from web scraping, let’s see an example of web scraping. We will try to get the title of the StackOverflow website with our Python script.
Here is the code:
from bs4 import BeautifulSoup as bs
import requests
url = "https://www.stackoverflow.com/"
html_content = requests.get(url).text
soup = bs(html_content, "html.parser")
if soup.find("title").text is not None:
print(soup.find("title").text)
Output:
Stack Overflow - Where Developers Learn, Share, & Build Careers
Here, you will notice that the if
condition we used is is not None
to ensure we’re calling an existing method. Now, it will not show any error such as the AttributeError
.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python