How to Solve AttributeError: 'Nonetype' Object Has No Attribute 'Group' in Python
-
Cause of
AttributeError: 'NoneType' object has no attribute 'group'
in Python -
Use
try-except
to SolveAttributeError: 'NoneType' object has no attribute 'group'
in Python -
Use
if-else
to SolveAttributeError: 'NoneType' object has no attribute 'group'
in Python
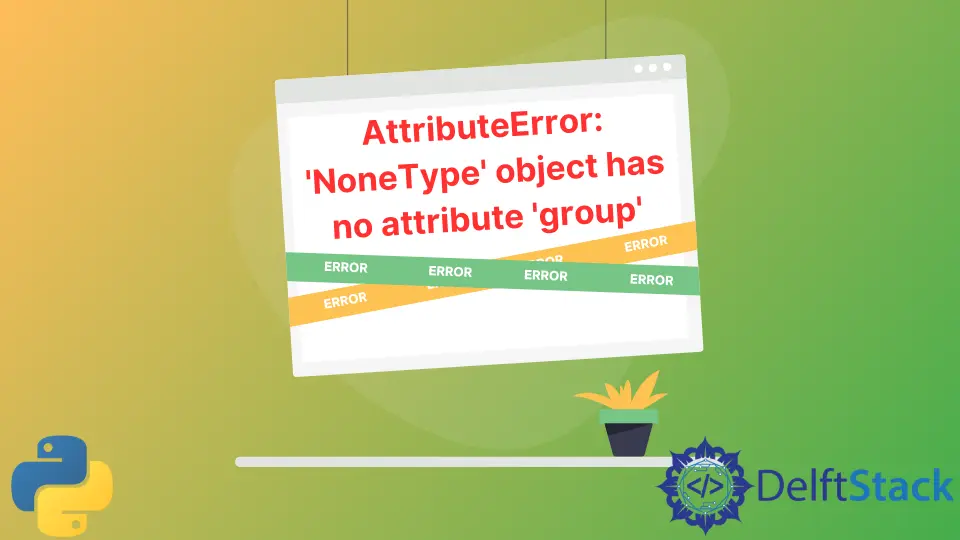
Python regular expression (regex) matches and extracts a string of special characters or patterns. In Python, the regex AttributeError: 'NoneType' object has no attribute 'group'
arises when our regular expression fails to match the specified string.
In this article, we will take a look at possible solutions to this type of error.
Cause of AttributeError: 'NoneType' object has no attribute 'group'
in Python
Whenever we define a class or data type, we have access to the attributes associated with that class. But suppose we access the attributes or properties of an object not possessed by the class we defined.
In that case, we encounter the AttributeError
saying the 'NoneType' object has no attribute 'group'
. The NoneType
refers to the object containing the None
value.
This type of error also occurs in a case when we set a variable initially to none. The following program is for searching for the uppercase F
at the beginning of a word.
Example Code:
# Python 3.x
import re
a = "programmig is Fun"
for i in a.split():
b = re.match(r"\bF\w+", i)
print(b.group())
Output:
#Python 3.x
AttributeError Traceback (most recent call last)
C:\Users\LAIQSH~1\AppData\Local\Temp/ipykernel_2368/987386650.py in <module>
3 for i in a.split():
4 b=re.match(r"\bF\w+", i)
----> 5 print(b.group())
AttributeError: 'NoneType' object has no attribute 'group'
This error is raised because the regular expression cannot match the specified letter in the string in the first iteration. Thus, when we access group()
, the compiler shows an AttributeError
because it belongs to the object of type None.
Use try-except
to Solve AttributeError: 'NoneType' object has no attribute 'group'
in Python
One method to eliminate this error is using exception handling in your code. In this way, the except
block will handle the error.
Now consider the previous program, and we will add the try-except
block as follows.
Example Code:
# Python 3.x
import re
a = "programmig is Fun"
for i in a.split():
b = re.match(r"\bF\w+", i)
try:
print(b.group())
except AttributeError:
continue
Output:
#Python 3.x
Fun
Now we see that our program works fine. The keyword continue
is used here to skip where b
returns none, jumps to the next iteration, and searches for a word that begins with F
.
Hence, the term Fun
prints in the output.
Use if-else
to Solve AttributeError: 'NoneType' object has no attribute 'group'
in Python
Another simple solution to avoid the 'NoneType' object has no attribute 'group'
error is to use the if-else
statement in your program. The following program checks the numbers in the string ranging from 1 to 5.
Since there is no number to match the regular expression, it results in an AttributeError
. But using the if-else
block, we can avoid the error.
If the condition is not satisfied, the statement in the else
block executes when no matches are found.
# Python 3.x
import re
a = "foo bar 678 baz"
x = r".* ([1-5]+) .*"
matches = re.match(x, a)
if matches:
print(matches.group(1))
else:
print("No matches!")
Output:
#Python 3.x
No matches!
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python