How to Fix AttributeError: 'generator' Object Has No Attribute 'next' in Python
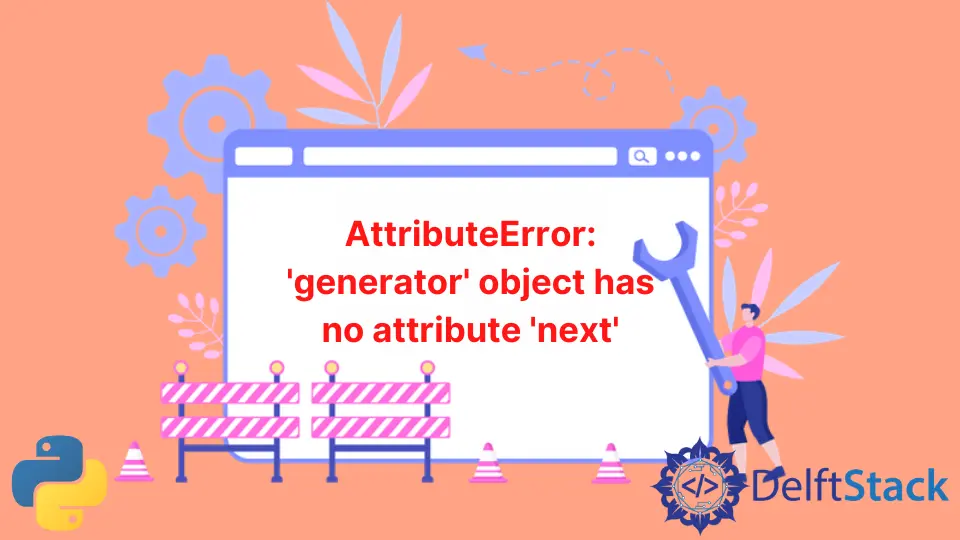
Attributes are values related to an object or a class. The AttributeError
is raised in Python when you call an attribute of an object whose type is not supported by the method.
For example, using the split()
method on an int object returns an AttributeError
because int objects do not support the split()
method.
In Python 3, there is no .next
method attached to the iterator. As a result, you will get an AttributeError
when you try to use the .next
method on the generator
object.
This tutorial will teach you to fix the AttributeError: 'generator' object has no attribute 'next'
in Python.
Fix the AttributeError: 'generator' object has no attribute 'next'
Error in Python
Here is an example of AttributeError
while using the yield
statement in Python 3.
def get_data(n):
for i in range(n):
yield i
a = get_data(20)
for i in range(10):
print(a.next())
Output:
Traceback (most recent call last):
File "c:\Users\rhntm\myscript.py", line 6, in <module>
print(a.next())
AttributeError: 'generator' object has no attribute 'next'
As you can see, there is an AttributeError
in line 6 containing the code print(seq.next())
. It is because we have used the .next
method to get the next item from the iterator.
The .next
method is replaced by the built-in function next()
in Python 3. You can fix this error using the next
function, as shown below.
def get_data(n):
for i in range(n):
yield i
a = get_data(20)
for i in range(10):
print(next(a))
Output:
0
1
2
3
4
5
6
7
8
9
In Python 2, you can use the .next
method, but Python 3 throws an exception, AttributeError
.
Now you know how to solve the error 'generator' object has no attribute 'next'
in Python. We hope you find this solution helpful.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python