How to Solve Python AttributeError: '_io.TextIOWrapper' Object Has No Attribute 'Split'
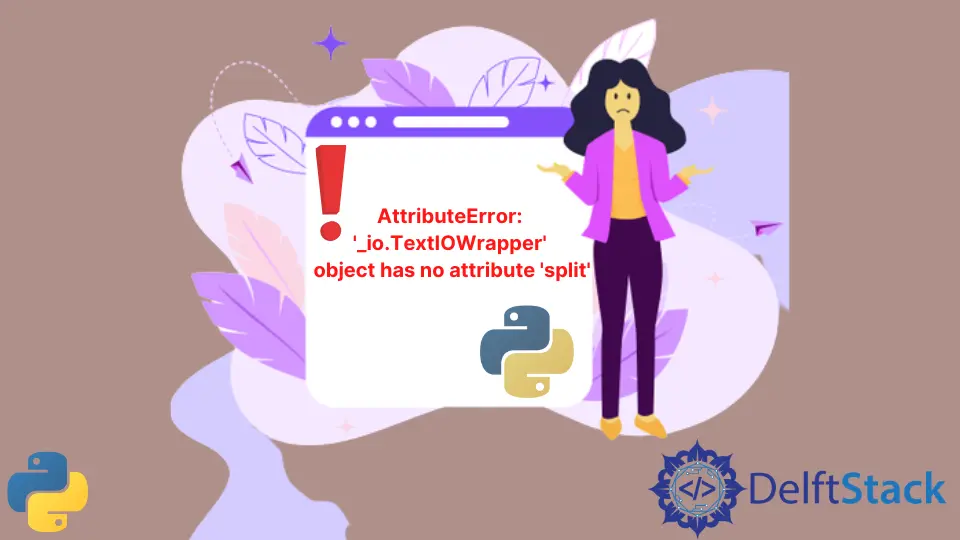
Attributes are values related to an object or a class. A Python AttributeError
occurs when you call an attribute of an object whose type is not supported by the method.
For example, using the split()
method on a _io.TextIOWrapper
returns an AttributeError
because the _io.TextIOWrapper
objects do not support the split()
method.
This tutorial will teach you to fix the AttributeError: '_io.TextIOWrapper' object has no attribute 'split'
in Python.
Fix the AttributeError: '_io.TextIOWrapper' object has no attribute 'split'
Error in Python
The following command uses the split()
method on an open file object.
f = open("test.txt")
f.split()
Output:
It returns the AttributeError
because the split()
method is not an attribute of the class _io.TextIOWrapper
. The String
class provides the split()
method to split the string into a list.
You can fix this error by using the for
loop.
f = open("test.txt")
for line in f:
line.split()
It does not return any error because each line in a file object is a string.
You can also use the methods available in the class _io.TextIOWrapper
to convert a file object to a string.
read()
- This method reads the file content and returns them as a string.readline()
- It reads a single line in a file and returns it as a string.readlines()
- This method helps to read the file content line by line and return them as lists of strings.
Then you can call the split()
method without getting an AttributeError
.
f = open("test.txt")
str = f.read()
str.split()
Now you know how to solve AttributeError
in Python. We hope you found this article helpful.
Related Article - Python AttributeError
- How to Fix AttributeError: Int Object Has No Attribute
- How to Fix AttributeError: __Exit__ in Python
- How to Fix AttributeError: 'NoneType' Object Has No Attribute 'Text' in Python
- How to Solve Python AttributeError: _csv.reader Object Has No Attribute Next
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python