How to Solve Python AttributeError: _csv.reader Object Has No Attribute Next
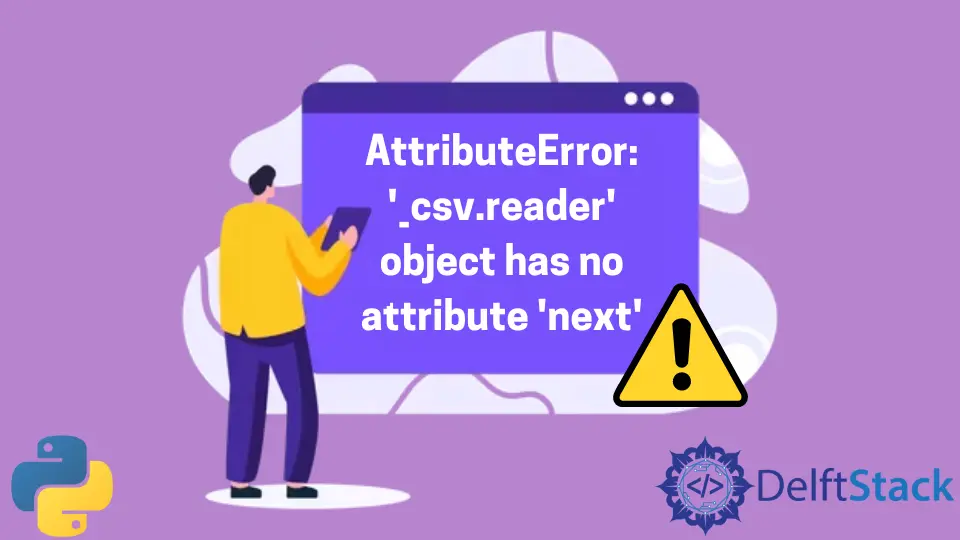
The CSV format is one of the most used formats in spreadsheets and databases. The Python language has the csv
module that provides classes to read and write data in CSV format.
Attributes are values that are related to an object or a class. An AttributeError
occurs in Python when you call an attribute of an object whose type is not supported by the method.
For instance, using the split()
method on a file object returns an AttributeError
because the file objects do not support the split()
method.
This tutorial will teach you to fix the AttributeError: '_csv.reader' object has no attribute 'next'
in Python.
Fix the AttributeError: '_csv.reader' object has no attribute 'next'
Error in Python
The csv.reader
object is an iterator. The next()
method is available in the csv.reader
object and returns the next row of the iterable object.
import csv
with open(csvfile) as f:
reader = csv.reader(f, delimiter=",", quotechar='"', skipinitialspace=True)
header = reader.next()
f.close()
Output:
line 5, in <module>
header = reader.next()
AttributeError: '_csv.reader' object has no attribute 'next'
But in Python 3, you have to use the built-in function next(reader)
instead of the reader.next()
method.
import csv
with open(csvfile) as f:
reader = csv.reader(f, delimiter=",", quotechar='"', skipinitialspace=True)
header = next(reader)
f.close()
With this, the AttributeError
should be solved in Python. We hope you found this article helpful.
Related Article - Python AttributeError
- How to Fix AttributeError: Int Object Has No Attribute
- How to Fix AttributeError: __Exit__ in Python
- How to Fix AttributeError: 'NoneType' Object Has No Attribute 'Text' in Python
- How to Solve Python AttributeError: '_io.TextIOWrapper' Object Has No Attribute 'Split'
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python