Assignment Operators in Python
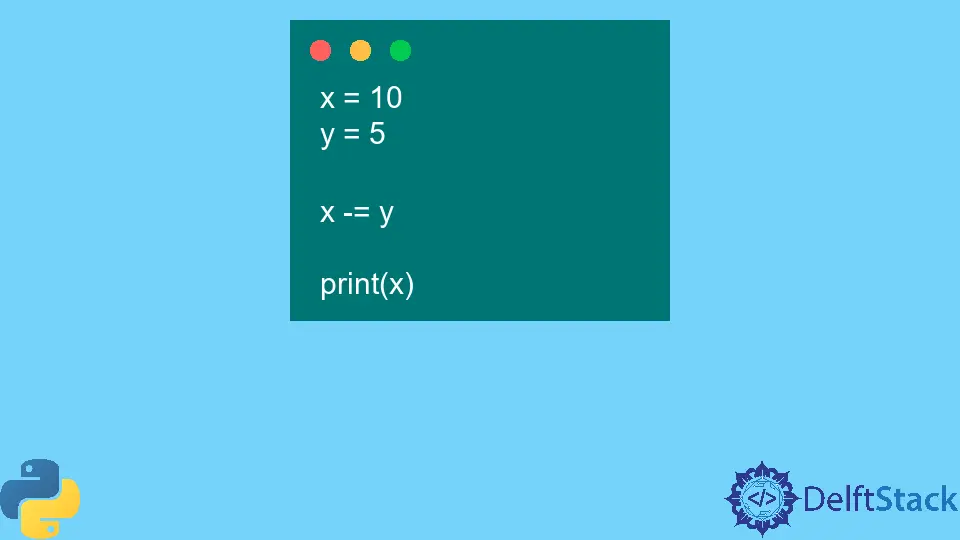
There are lots of times in programming when we have to assign values to various variables. In Python, there are different assignment operators for doing these kinds of tasks. You can use two types of assignment operators in Python: simple assignment operators like =
, and compound assignment operators like +=
and -=
.
This tutorial will tell what the -=
assignment operator is in Python.
the -=
Operator in Python
The -=
operator is a decremental assignment operator in Python. It subtracts the operand on the right side from the operator on the left side and finally assigns the resultant difference to the operand on the left side.
So, we can say that c -= 10
is similar to c = c - 10
.
Now let us see how we can use the -=
operator in Python.
x = 10
y = 5
x -= y
print(x)
Output:
5
Here, the x -= y
statement means x = x - y
i.e., x = 10 - 5
, which is equal to 5
.
Just like the -=
operator, there are many different types of compound assignment operators like +=
, *=
, /=
, &=
, and |=
that help in carrying out all the basic kinds of arithmetic operations, boolean operations, and others.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn