How to Solve IndexError: Arrays Used as Indices Must Be of Integer (Or Boolean) Type
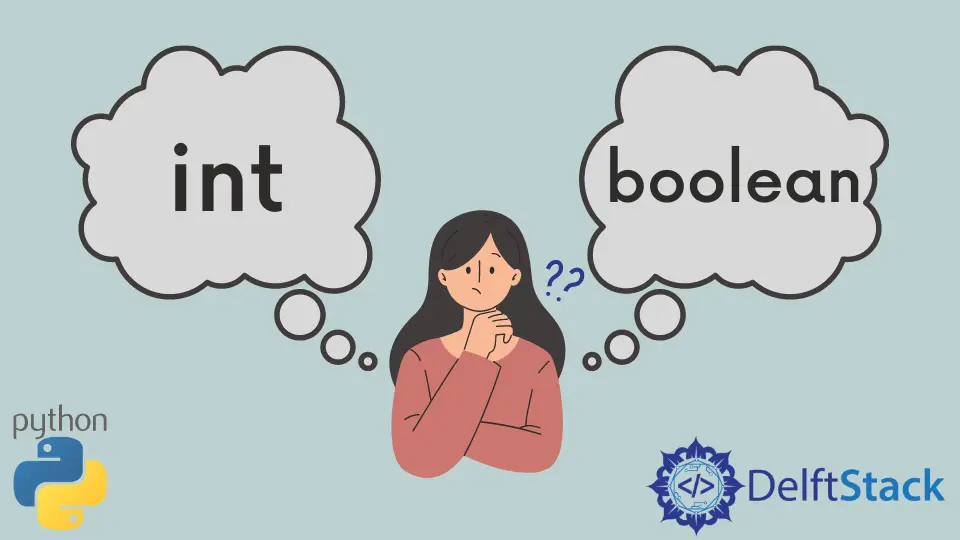
When working with Numpy arrays in Python, you might experience different error messages dealing with Index or Type issues. In these many error types, IndexError: arrays used as indices must be of integer (or boolean) type
can be tricky.
When we face IndexError
error messages, we use the wrong Type. In this case, we were supposed to use Integer
or Boolean
, but the array index receives another data type (string or float).
In this article, we will explain how to deal with IndexError: arrays used as indices must be of integer (or boolean) type
error messages when working with numbers in Numpy.
Use astype()
to Solve IndexError: arrays used as indices must be of integer (or boolean) type
in Numpy
Numpy only works with two types, Integer or Boolean. Therefore, if there is a type it doesn’t understand, it will throw an error.
Let’s recreate the error message to understand this error message better. To recreate the error message, we need to generate two Numpy arrays, index
and array
, extract values from index
, and use the extracted values to access the values in array
.
For the extracted values, we will use the first column values.
import numpy as np
index = np.array([[0, 1, 2.1], [1, 2, 3.4]])
array = np.array([[1, 2, 3], [4, 5, 6]])
indices = index[:, 0]
print(array[indices])
Output:
Traceback (most recent call last):
File "temp.py", line 7, in <module>
print(array[indices])
IndexError: arrays used as indices must be of integer (or boolean) type
From the IndexError: arrays used as indices must be of integer (or boolean) type
error message, we know the problem stems from the print(array[indices])
section.
Since we know that it is syntactically correct, we know that the issue we are looking for is present in what we are parsing to the array
binding. That brings us to the indices
binding.
From what we know from the error message, the element in the indices
binding might not be integer
or Boolean
. The dtype
property is useful to check the type of elements within indices
.
print(indices.dtype)
Output:
float64
Now, that confirms the cause of the issue we are facing. The values we pass to the indices of the array
binding are float64
instead of Boolean
.
To solve this, we need to convert the values in indices
to Integer
or Boolean
. It makes more sense to convert them to Integer
.
Converting them to Boolean
might be useful another time.
The astype()
method helps modify the dtype
property of a Numpy array. To modify the dtype
of the indices
binding, we can use the below.
indices = index[:, 0].astype(int)
We get the below if we check for the dtype
property using the indices.dtype
expression.
int32
Now, our code becomes:
import numpy as np
index = np.array([[0, 1, 2.1], [1, 2, 3.4]])
array = np.array([[1, 2, 3], [4, 5, 6]])
indices = index[:, 0].astype(int)
print(array[indices])
Output:
[[1 2 3]
[4 5 6]]
We could have converted the values of indices
to Boolean
. Let’s experiment with that.
To do so, we have a Numpy array with two Booleans.
indices = index[:, 0].astype(bool)
print(indices)
Output:
[[ 7 9 11]]
That’s because it processes only the True
value.
Therefore, when you face an IndexError: arrays used as indices must be of integer (or boolean) type
error message, know there is a wrong dtype
somewhere. Trace your code, and convert the necessary values.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python