How to Append Values to a Set in Python
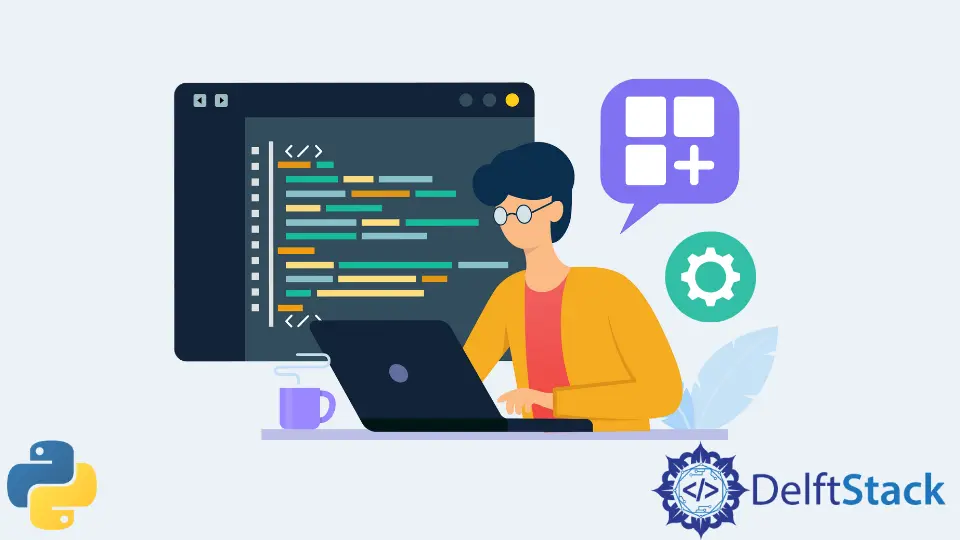
When working with sets in Python, you might find yourself needing to add new elements dynamically. Sets are unique collections that do not allow duplicate entries, making them perfect for scenarios where you want to ensure that your data remains distinct.
In this article, we will explore three primary methods for appending values to a set in Python: the add()
function, the update()
function, and the |=
operator. Each method has its unique advantages, and understanding them will enhance your coding skills and efficiency. So, let’s dive in and learn how to effectively manage sets in Python!
Using the add()
Function
The add()
method is one of the simplest ways to append a single value to a set. It allows you to introduce a new element while ensuring that no duplicates are created. If the element already exists in the set, the set will remain unchanged. This method is particularly useful when you want to add one item at a time.
Here’s how you can use the add()
method:
my_set = {1, 2, 3}
my_set.add(4)
print(my_set)
Output:
{1, 2, 3, 4}
In this example, we start with a set containing the numbers 1, 2, and 3. By using the add()
function, we append the number 4 to the set. The output confirms that 4 has been successfully added. If we attempted to add 2 again using the same method, the set would remain unchanged:
my_set.add(2)
print(my_set)
Output:
{1, 2, 3, 4}
The add()
method is straightforward and efficient for adding single elements. However, if you need to add multiple elements simultaneously, this method may not be the best choice. In such cases, you may want to explore the update()
method.
Using the update()
Function
The update()
method lets you append multiple values to a set at once. This function can take an iterable, such as a list or another set, and adds each element from the iterable to the target set. This is a powerful way to expand your set without having to call the add()
method multiple times.
Here’s an example of how to use the update()
method:
my_set = {1, 2, 3}
my_set.update([4, 5, 6])
print(my_set)
Output:
{1, 2, 3, 4, 5, 6}
In this code snippet, we start with a set containing the numbers 1, 2, and 3. We then use the update()
function to append the elements 4, 5, and 6 from a list. The output shows that all three new elements have been added to the set.
You can also use other iterables, such as sets or tuples, with the update()
method. For instance:
my_set.update({7, 8})
print(my_set)
Output:
{1, 2, 3, 4, 5, 6, 7, 8}
The update()
method is efficient for adding multiple values and is especially useful when you have a collection of items to include in your set. Keep in mind that, like the add()
method, update()
will not add duplicates, ensuring your set remains unique.
Using the |= Operator
The |=
operator is another efficient way to append multiple values to a set. This operator performs an in-place union of the set with another iterable, effectively adding all unique elements from the iterable to the original set. It’s a concise and elegant way to achieve the same result as the update()
method.
Here’s how to use the |=
operator:
my_set = {1, 2, 3}
my_set |= {4, 5, 6}
print(my_set)
Output:
{1, 2, 3, 4, 5, 6}
In this example, we start with a set containing 1, 2, and 3. By using the |=
operator, we append the elements 4, 5, and 6 from another set. The output shows that the new elements have been successfully added.
This operator can also be used with other iterables, such as lists or tuples, and it works similarly to the update()
method. For instance:
my_set |= [7, 8]
print(my_set)
Output:
{1, 2, 3, 4, 5, 6, 7, 8}
Using the |=
operator is a great way to keep your code clean and concise. It’s especially handy when you want to add multiple elements without explicitly calling a method like update()
.
Conclusion
In conclusion, appending values to a set in Python can be accomplished through several straightforward methods. The add()
function is perfect for adding single elements, while the update()
function and the |=
operator allow for the efficient addition of multiple elements. Understanding these methods will not only enhance your coding skills but also improve your ability to manage data effectively. As you continue to explore Python, remember that sets are a powerful tool for maintaining unique collections, and mastering these methods will serve you well in your programming journey.
FAQ
- What is a set in Python?
A set is a collection type in Python that stores unique elements, meaning no duplicates are allowed.
-
Can I add duplicate values to a set using the add() method?
No, theadd()
method will not add a duplicate value to a set; the set will remain unchanged. -
How does the update() method differ from the add() method?
Theupdate()
method allows you to add multiple elements at once, whileadd()
is used for a single element. -
What happens if I try to add a list to a set?
You cannot directly add a list to a set, but you can use theupdate()
method to append its elements. -
Is the |= operator the same as the update() method?
Yes, the|=
operator performs an in-place union of a set with another iterable, similar to theupdate()
method.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn