How to Append One String to Another in Python
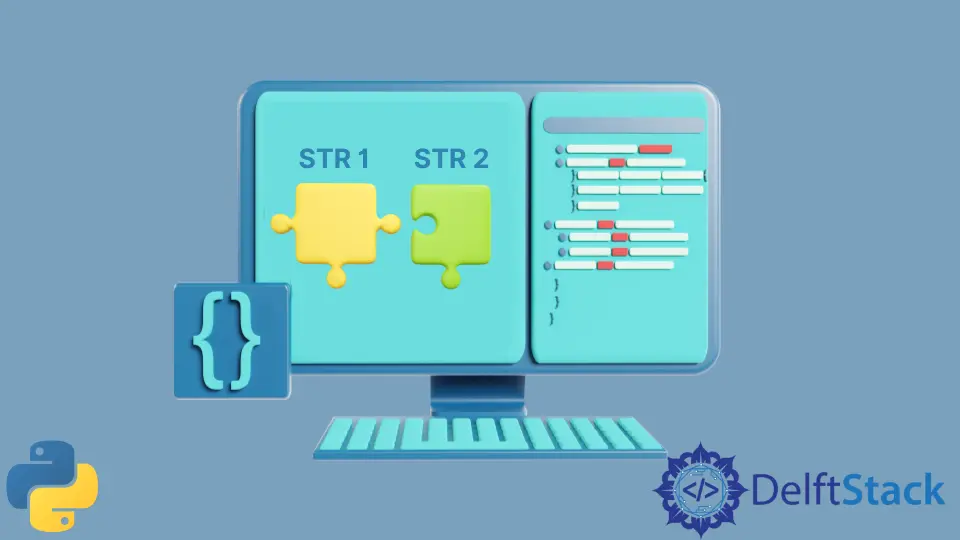
Appending one string to another in Python is a fundamental skill every programmer should master. Whether you’re a beginner or an experienced developer, understanding how to manipulate strings effectively can enhance your coding efficiency and make your programs more dynamic. In Python, there are two primary methods to achieve this: using the +
operator and utilizing the str.join()
function. Each method has its unique advantages and use cases, making them suitable for different scenarios.
In this article, we will explore both methods in detail, providing clear examples and explanations to help you grasp the concepts easily. Let’s dive into the world of string manipulation in Python!
Using the + Operator
The +
operator is one of the simplest and most intuitive ways to append strings in Python. When you use this operator, Python concatenates the two strings and returns a new string. This method is straightforward and perfect for quick operations or when you’re dealing with a small number of strings.
Here’s how you can use the +
operator to append strings:
string1 = "Hello"
string2 = "World"
result = string1 + " " + string2
print(result)
Output:
Hello World
In this example, we start with two strings: string1
and string2
. By using the +
operator, we concatenate them with a space in between. The result is a new string, “Hello World”, which we then print to the console.
While using the +
operator is quite efficient for a small number of strings, it may not be the best choice for larger datasets or multiple concatenations. This is because each use of the +
operator creates a new string, which can lead to increased memory usage and slower performance. As a result, if you find yourself appending strings in a loop or combining many strings, you might want to consider other methods.
Using the str.join() Function
Another effective way to append strings in Python is through the str.join()
function. This method is particularly useful when you need to concatenate multiple strings, as it allows you to specify a delimiter between them. The join()
function is both efficient and elegant, making it a popular choice among Python developers.
Here’s a quick example of how to use str.join()
:
strings = ["Hello", "World", "from", "Python"]
result = " ".join(strings)
print(result)
Output:
Hello World from Python
In this example, we have a list of strings that we want to concatenate. By calling the join()
method on a space string, we can easily combine all the strings in the list with spaces in between. The result is “Hello World from Python”, which we print to the console.
The str.join()
method is particularly advantageous when dealing with larger datasets or when you need to append many strings. It performs better than using the +
operator in such cases because it constructs the final string in a single operation, reducing memory overhead and improving performance. Additionally, you can easily change the delimiter to any character or string, giving you greater flexibility in how your final output appears.
Conclusion
Appending strings in Python is a crucial skill that can significantly enhance your programming capabilities. Whether you choose to use the +
operator for simple concatenations or the str.join()
function for more complex scenarios, understanding both methods will enable you to manipulate strings effectively. As you continue your journey in Python programming, remember to consider the context of your task to choose the most efficient approach. Happy coding!
FAQ
-
What is the difference between the + operator and str.join()?
The + operator is best for simple concatenations, while str.join() is more efficient for combining multiple strings. -
Can I use str.join() with non-string elements?
No, all elements must be strings. You can convert non-string elements to strings before using join(). -
Which method is faster for appending strings in a loop?
str.join() is generally faster and more memory-efficient for appending strings in a loop. -
Can I use str.join() with a list of strings?
Yes, str.join() works perfectly with lists of strings. -
Is it possible to append strings without any delimiter?
Yes, you can use an empty string as the delimiter in str.join() to concatenate without spaces.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn