How to Add a List to a Set in Python
- Understanding Sets and Lists in Python
-
Method 1: Using the
update()
Method - Method 2: Using a Loop
- Method 3: Using Set Comprehension
- Conclusion
- FAQ
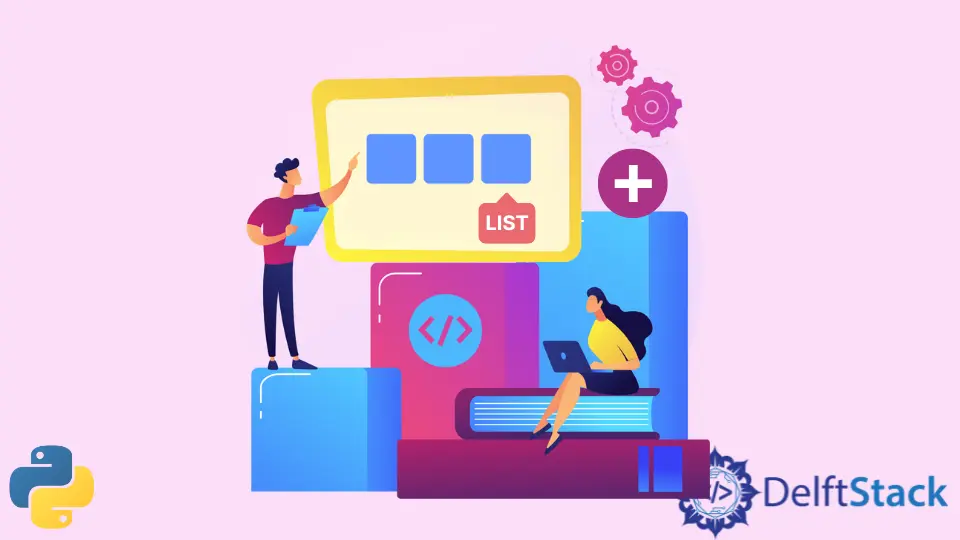
Adding values from a list to a set in Python is a common task that can enhance your data manipulation capabilities. Sets are unique collections that automatically handle duplicates, making them ideal for various applications.
In this tutorial, we will explore several methods to add list values to a set, providing you with practical code examples along the way. Whether you’re looking to combine data structures or simply want to ensure uniqueness in your collections, this guide will equip you with the knowledge to do so effectively. So, let’s dive in and discover how to seamlessly integrate lists into sets in Python!
Understanding Sets and Lists in Python
Before we get into the methods of adding a list to a set, it’s essential to understand the fundamental differences between these two data structures. A list in Python is an ordered collection that allows duplicates, while a set is an unordered collection that does not allow duplicate values. This property of sets makes them particularly useful when you need to ensure that all elements are unique.
When working with sets, you can easily add elements using methods like add()
and update()
. The add()
method is used to add a single element, while update()
can take an iterable, such as a list, and add all its elements to the set. With this understanding, let’s explore the various methods to add a list to a set.
Method 1: Using the update()
Method
One of the simplest and most efficient ways to add a list to a set in Python is by using the update()
method. This method allows you to add multiple elements to a set at once, making it perfect for our use case.
Here’s how you can do it:
my_set = {1, 2, 3}
my_list = [3, 4, 5]
my_set.update(my_list)
print(my_set)
Output:
{1, 2, 3, 4, 5}
In this example, we start with a set containing the numbers 1, 2, and 3. We then define a list with the values 3, 4, and 5. When we call my_set.update(my_list)
, the method adds all the elements from my_list
to my_set
. Since sets do not allow duplicates, the value 3 is only included once. As a result, the final set contains the unique values 1, 2, 3, 4, and 5.
Using update()
is particularly advantageous when you want to combine multiple collections while ensuring that you don’t have any duplicates in your final set. It’s a clean and efficient way to achieve this.
Method 2: Using a Loop
Another method to add elements from a list to a set is by using a simple loop. This approach allows for more control over how elements are added, and you can implement additional logic if needed.
Here’s a code snippet illustrating this method:
my_set = {1, 2, 3}
my_list = [3, 4, 5]
for item in my_list:
my_set.add(item)
print(my_set)
Output:
{1, 2, 3, 4, 5}
In this case, we again start with a set containing 1, 2, and 3. We then iterate over each element in my_list
. For each element, we use the add()
method to insert it into my_set
. This method works similarly to update()
, ensuring that duplicates are not added. The end result is the same: our set now contains the unique values 1, 2, 3, 4, and 5.
Using a loop can be particularly useful if you need to perform additional checks or transformations on the elements before adding them to the set, giving you greater flexibility in your code.
Method 3: Using Set Comprehension
Set comprehension is another elegant way to add values from a list to a set. This method allows you to create a new set in a concise manner, leveraging the power of Python’s comprehension syntax.
Here’s how you can use set comprehension:
my_list = [3, 4, 5]
my_set = {x for x in my_list}
print(my_set)
Output:
{3, 4, 5}
In this example, we create a new set directly from my_list
using set comprehension. The expression {x for x in my_list}
iterates over each item in my_list
and adds it to the new set. This method is particularly useful when you want to create a set from a list while applying some transformation or filtering criteria.
If you want to combine this with an existing set, you can simply use the update()
method afterward:
existing_set = {1, 2}
existing_set.update({x for x in my_list})
print(existing_set)
Output:
{1, 2, 3, 4, 5}
Here, we first create a set from my_list
and then update existing_set
with the new values. This method is not only concise but also very readable, making it a great choice for Python developers.
Conclusion
Adding a list to a set in Python can be accomplished in several ways, each with its own advantages. Whether you prefer the simplicity of the update()
method, the control offered by a loop, or the elegance of set comprehension, Python provides you with the tools to effectively manage your data structures. By understanding these methods, you can ensure that your sets remain unique and your code stays clean and efficient. So, the next time you need to combine a list with a set, you’ll know exactly how to do it!
FAQ
-
What happens if I add duplicate values from a list to a set?
A set will only keep unique values, so any duplicates from the list will be ignored. -
Can I add a list of strings to a set in Python?
Yes, you can add a list of strings to a set using the same methods outlined in this tutorial. -
Is there a performance difference between using
update()
and a loop?
Generally,update()
is more efficient for adding multiple items at once, while a loop gives you more control. -
Can I add other data types to a set?
Yes, sets can contain elements of different data types, including integers, strings, and tuples. -
What is the difference between
add()
andupdate()
methods?
Theadd()
method adds a single element to a set, whileupdate()
can add multiple elements from an iterable.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python