__init__.py in Python
Shivam Arora
Oct 10, 2023
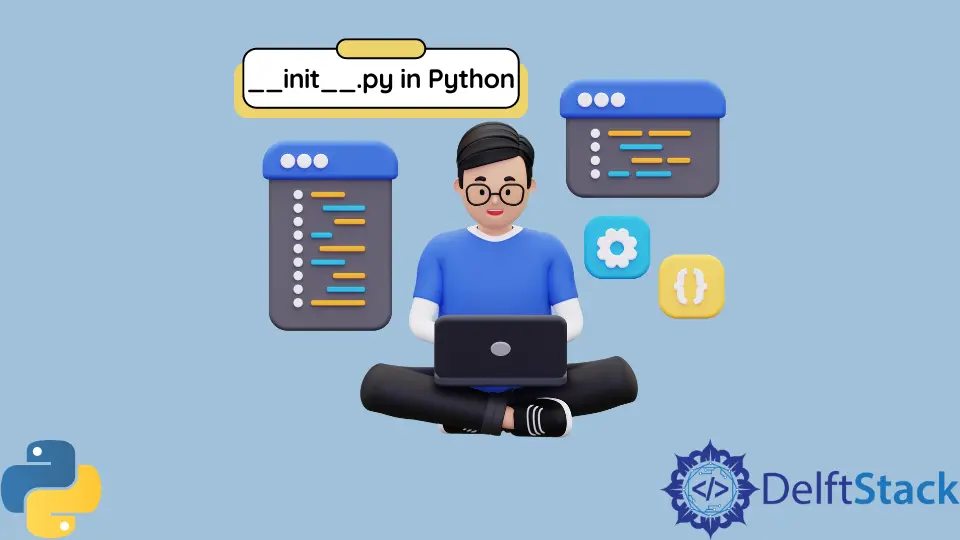
In Python, there are two types of packages named regular packages and namespace packages. The Regular packages exist in Python 3.2 and previous versions. These packages are used as a directory that contains the __init__.py
file, which is implicitly called or executed.
The __init__.py
file in Python lets the Interpreter knows that a directory contains a Python code in the module. This file can have the same code as any other Python module.
Files named as __init__.py
are used to flag directories as Python packages.
For example,
mydir / spam / __init__.py
mydir / spam / module.py
If this file is removed, Python can not search for submodules in the directory, leading to failure in importing the module.
Main Reasons to Use __init__.py
in Python
- Using
__init__.py
will allow other users not to know the exact locations of the functions in the package.
your_package/
__init__.py
file1.py
file2.py
...
fileN.py
- Using
__init__.py
will help in initializing some details like logging at the top.
import logging.config
logging.config.dictConfig(Your_logging_config)
__init__.py
helps in easing the import of files. A functionfn()
can be imported from a filefilename.py
when it is in a package.
from filename import fn()