How to Change Tkinter Window Title
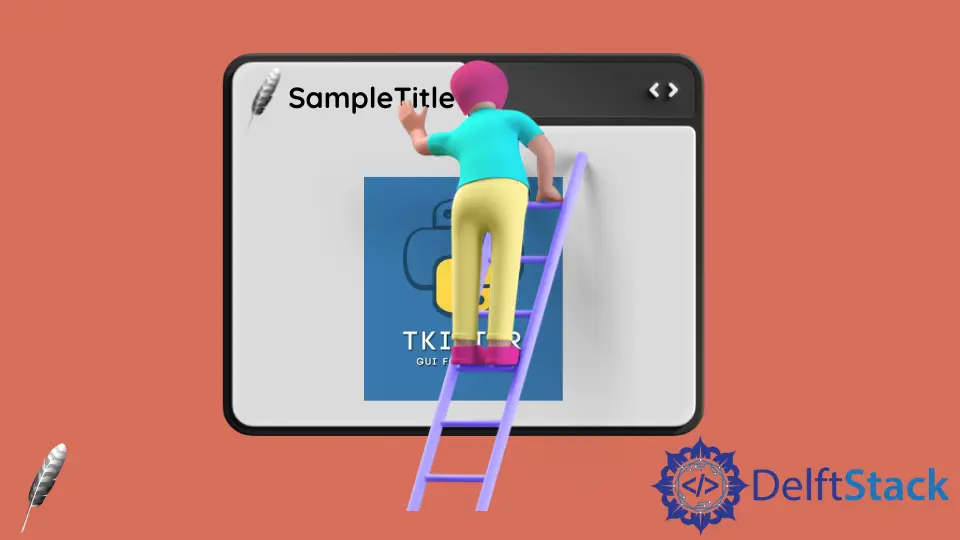
In this tutorial, we will learn how to change the title bar of a tkinter
window.
The title in tkinter
refers to a name assigned to the application window. It is mostly found on the top of the application. For this, we can use the title()
function.
We create a widget object using the Tk()
function and use the title()
function to add a title to this window.
For example,
from tkinter import *
ws = Tk()
ws.title("Hello_World")
ws.geometry("400x300")
ws.mainloop()
Output:
In the above example, we can see our window with the title Hello_World
.
If we want to change the text style, font size, or the color of the title, then it can’t be done using tkinter
as the only purpose of tkinter
is to provide a name that has its own size and style by default.
If we want to set the title on the tkinter
frame, we have to use the function LabelFrame()
, which helps us set the title on the frame. This function takes the font size and the text to be displayed as the inputs. Also, we can change the color of it.
The code below demonstrates this.
from tkinter import *
ws = Tk()
ws.title(string="")
ws.geometry("400x300")
frame = LabelFrame(ws, text="Hello_World", bg="#f0f0f0", font=(30))
frame.pack(expand=True, fill=BOTH)
Button(frame, text="Submit").pack()
ws.mainloop()
Output: