How to Use a Timer in Tkinter
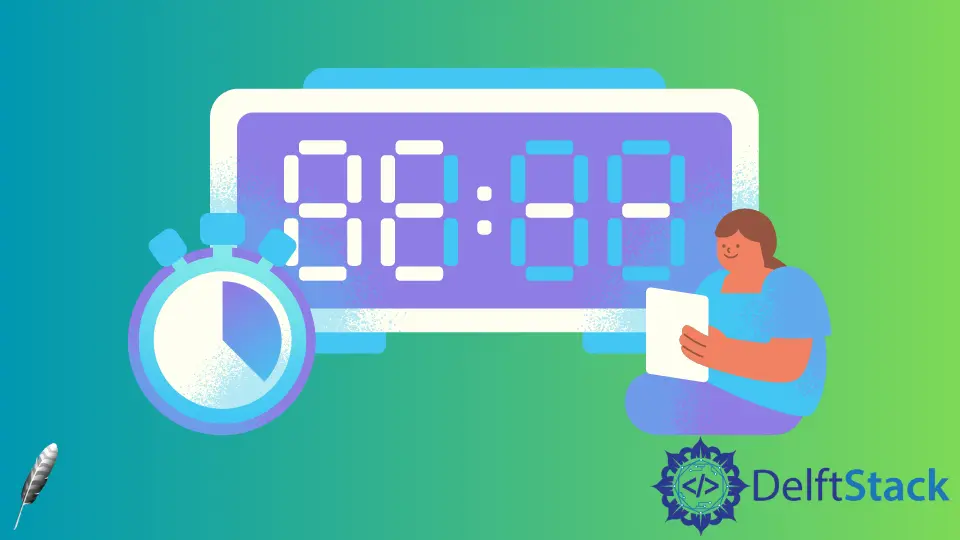
Tkinter root window has a dedicated method after
that calls a function after a given time period -
after(ms, func)
ms
is the interval in the unit of ms
,
func
is the called function name.
try:
import Tkinter as tk
except:
import tkinter as tk
import time
class Clock:
def __init__(self):
self.root = tk.Tk()
self.label = tk.Label(text="", font=("Helvetica", 48), fg="red")
self.label.pack()
self.update_clock()
self.root.mainloop()
def update_clock(self):
now = time.strftime("%H:%M:%S")
self.label.configure(text=now)
self.root.after(1000, self.update_clock)
app = Clock()
self.root.after(1000, self.update_clock)
calls the function itself after 1000
ms, therefore, update_clock()
function is executed at the interval of 1000
ms and displays the current time in the Tkinter label.
after
method is not guaranteed to call the function after the precise period, because it could be delayed if the application is busy, resulting from the fact that Tkinter is single-threaded.Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook