How to Set Window Icon in Tkinter
-
root.iconbitmap
to Set Window Icon -
tk.call('wm', 'Iconphoto', )
Method to Set Window Icon -
root.iconphoto
to Set Window Icon
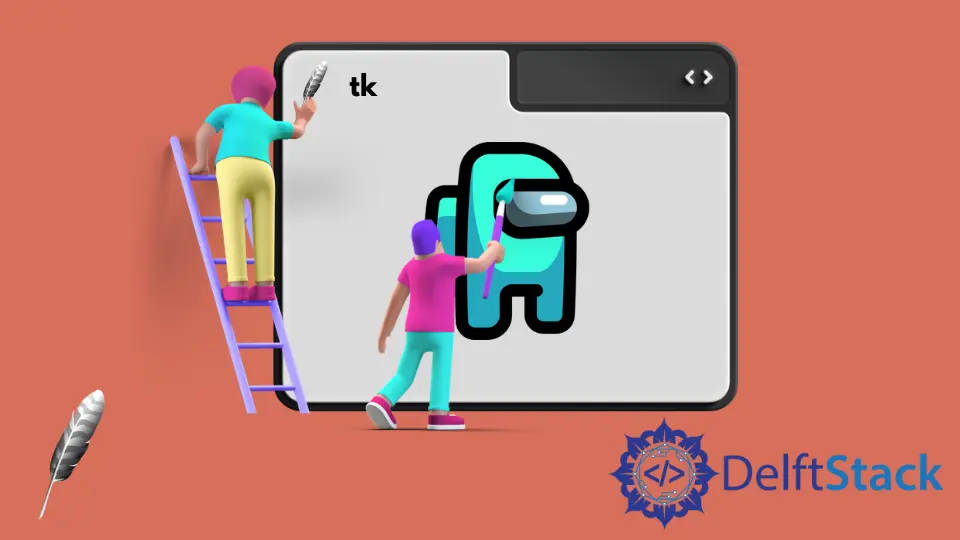
We will introduce methods to set the window icon in Tkinter.
root.iconbitmap
to set window iconroot.tk.call()
to set window iconroot.iconphoto
to set window icon
root.iconbitmap
to Set Window Icon
import tkinter as tk
root = tk.Tk()
root.iconbitmap("/path/to/ico/icon.ico")
root.mainloop()
iconbitmap(bitmap)
sets the icon of the window/frame widget to bitmap
. The bitmap
must be an ico
type, but not png
or jpg
type, otherwise, the image will not display as the icon.
The above image shows when the ico
type is used in iconbitmap
.
If you use png
type, the icon shown in the window will be blank,
tk.call('wm', 'Iconphoto', )
Method to Set Window Icon
import tkinter as tk
root = tk.Tk()
root.tk.call("wm", "iconphoto", root._w, tk.PhotoImage(file="/path/to/ico/icon.png"))
root.mainloop()
tk.call
method is the Tkinter interface to tcl
interpreter. We could run a tcl
command by using this call
method.
It is handy when the Tkinter wrapper couldn’t have access to some tcl/tk
features.
wm
communicates with window manager.
We need to set the image as tk.PhotoImage
but not the image itself, otherwise we will have a _tkinter.TclError
error.
root.iconphoto
to Set Window Icon
Another method to set window icon is to use root.iconphoto()
method that accepts more image types just as in tk.call('wm', 'iconphoto', )
.
import tkinter as tk
root = tk.Tk()
root.iconphoto(False, tk.PhotoImage(file="/path/to/ico/icon.png"))
root.mainloop()
Here, False
means this icon image applies only to this specific window but not future created toplevels
.
If True
is used, the icon image is applied to all future created toplevels
as well.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook