How to Set Text of Tkinter Text Widget With a Button
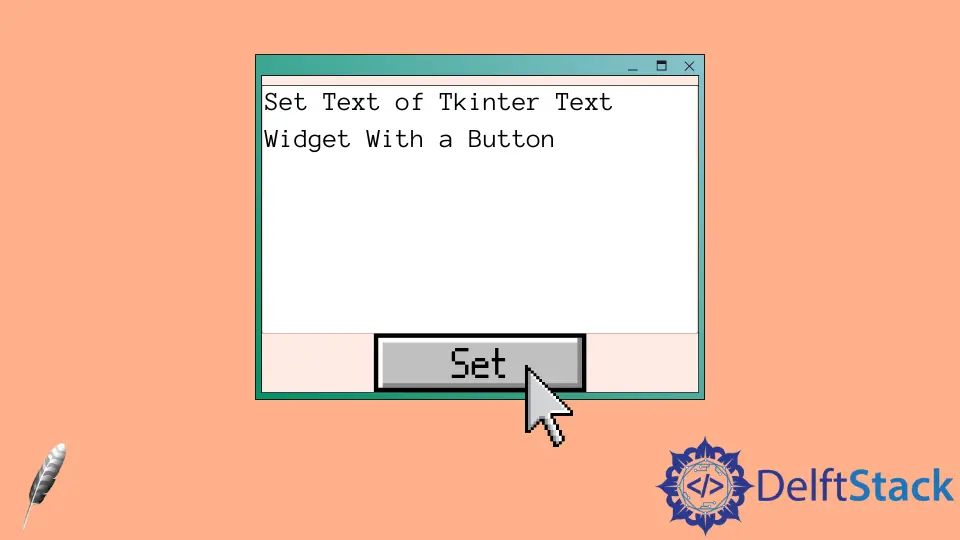
Tkinter Text
widget doesn’t have a dedicated set
method to set the content of the Text
. It needs to first delete the existing content and then insert the new content if we have to change the content completely.
This article will introduce how to set the Tkinter widget usinga Tkinter button.
Complete Working Codes to Set Text in Text
With delete
and insert
Methods
import tkinter as tk
root = tk.Tk()
root.geometry("400x240")
def setTextInput(text):
textExample.delete(1.0, "end")
textExample.insert(1.0, text)
textExample = tk.Text(root, height=10)
textExample.pack()
btnSet = tk.Button(
root, height=1, width=10, text="Set", command=lambda: setTextInput("new content")
)
btnSet.pack()
root.mainloop()
textExample.delete(1.0, "end")
delete
method of Text
deletes the specified range of characters in the Text
box, as introduced in the article of how to clear Tkinter Text
box.
1.0
is the first character and "end"
is the last character of the content in the Text
widget. Therefore, it deletes all the content inside the Text
box.
textExample.insert(1.0, text)
insert
method inserts the text at the specified position. In the above code, it inserts the text at the beginning.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook