How to Set the Default Text of Tkinter Entry Widget
-
insert
Method to Set the Default Text ofEntry
Widget -
Tkinter
StringVar
Method to Set the Default Text of TkinterEntry
Widget
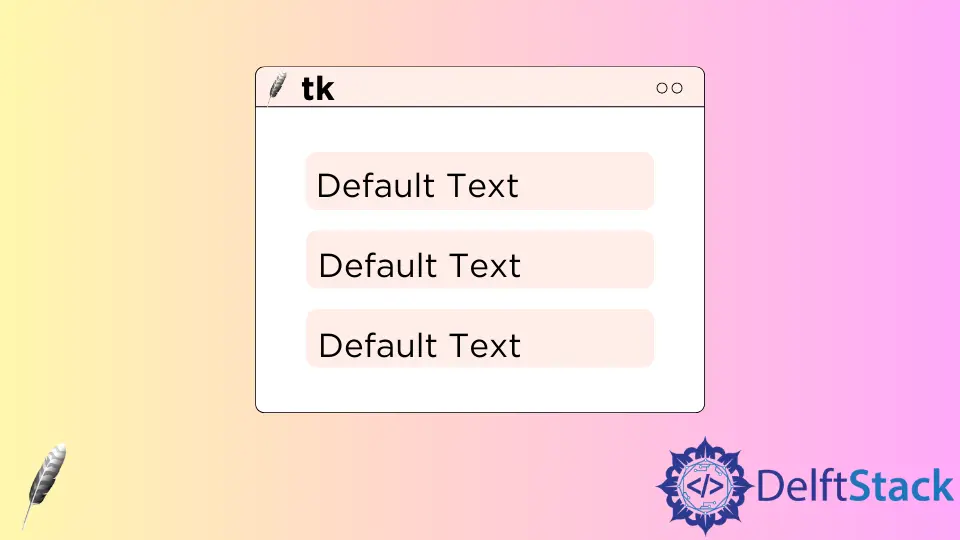
Tkinter has two methods to set the default text of Tkinter Entry widget.
- Tkinter
insert
Method - Tkinter
StringVar
Method
insert
Method to Set the Default Text of Entry
Widget
Tkinter Entry
widget doesn’t have a specific text
property to set the default text like text="example"
. It has insert
method to insert the text of the Entry
widget so that it could equivalently set the default text of Entry
if we call insert
method after the Entry
object is initialized.
Complete Working Codes to Set the Default Text of Entry
With insert
Methods
import tkinter as tk
root = tk.Tk()
root.geometry("200x100")
textExample = tk.Entry(root)
textExample.insert(0, "Default Text")
textExample.pack()
root.mainloop()
textExample.insert(0, "Default Text")
insert
method inserts the text at the specified position. 0
is the first character so that it inserts the Default Text
at the beginning.
Tkinter StringVar
Method to Set the Default Text of Tkinter Entry
Widget
textvariable
associates the content of the Entry
widget with a Tkinter StringVar
variable. It could set the StringVar
to set the default text of Entry
widget after the proper association is created.
Complete Working Codes to Set the Default Text in Entry
With textvariable
import tkinter as tk
root = tk.Tk()
root.geometry("200x100")
textEntry = tk.StringVar()
textEntry.set("Default Text")
textExample = tk.Entry(root, textvariable=textEntry)
textExample.pack()
root.mainloop()
textEntry = tk.StringVar()
textEntry.set("Default Text")
textExample = tk.Entry(root, textvariable=textEntry)
textEntry
is a StringVar
variable and it is associated with the text content of Entry
object by textvariable = textEntry
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook