How to Set Border of Tkinter Label Widget
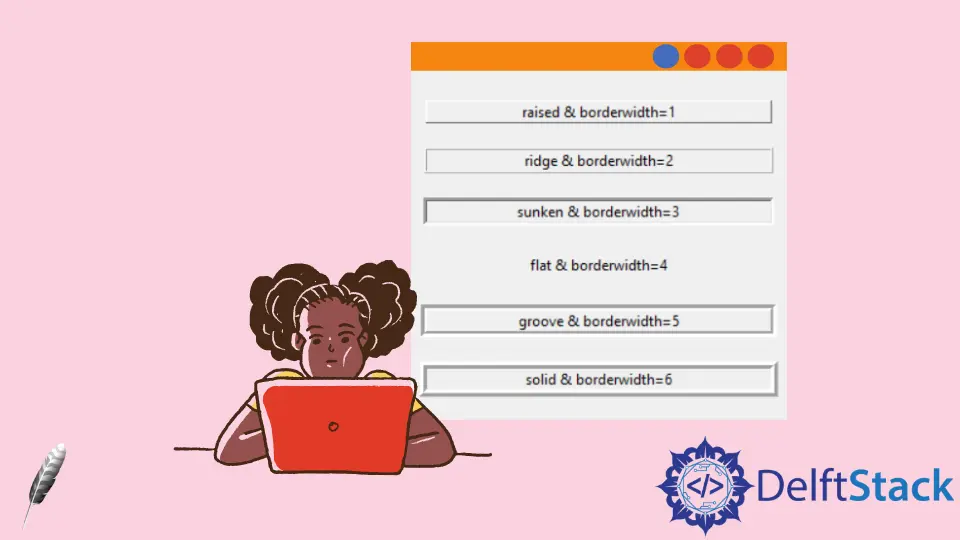
In this tutorial, we will introduce how to set the border of the Tkinter Label
.
Tkinter Label
doesn’t have the border by default as shown below.
You need to assign the borderwidth
option to add a border around Label
widget, and also assign the relief
option to be any option rather than flat
to make border visible.
tk.Label(app, borderwidth=3, relief="sunken", text="sunken & borderwidth=3")
It sets the borderwidth
as 3
and border decoration option relief
to be sunken
.
The below example shows the label order with different relief
options.
As you could see, the border is invisible when the relief
is flat
(default relief
value), even when the borderwidth
is set.
Complete Working Code Example
import tkinter as tk
app = tk.Tk()
labelExample1 = tk.Label(
app, borderwidth=1, width=40, relief="raised", text="raised & borderwidth=1"
)
labelExample2 = tk.Label(
app, borderwidth=2, width=40, relief="ridge", text="ridge & borderwidth=2"
)
labelExample3 = tk.Label(
app, borderwidth=3, width=40, relief="sunken", text="sunken & borderwidth=3"
)
labelExample4 = tk.Label(
app, borderwidth=4, width=40, relief="flat", text="flat & borderwidth=4"
)
labelExample5 = tk.Label(
app, borderwidth=5, width=40, relief="groove", text="groove & borderwidth=5"
)
labelExample6 = tk.Label(
app, borderwidth=6, width=40, relief="ridge", text="solid & borderwidth=6"
)
labelExample1.grid(column=0, row=0, padx=10, pady=10)
labelExample2.grid(column=0, row=1, padx=10, pady=10)
labelExample3.grid(column=0, row=2, padx=10, pady=10)
labelExample4.grid(column=0, row=3, padx=10, pady=10)
labelExample5.grid(column=0, row=4, padx=10, pady=10)
labelExample6.grid(column=0, row=5, padx=10, pady=10)
app.mainloop()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook