How to Get the Tkinter Label Text
-
cget
Method to Gettext
Option Value of TkinterLabel
-
Read Value of Key
text
of Label Object Dictionary to Get TkinterLabel
Text -
Use
StringVar
to Get the TkinterLabel
Text
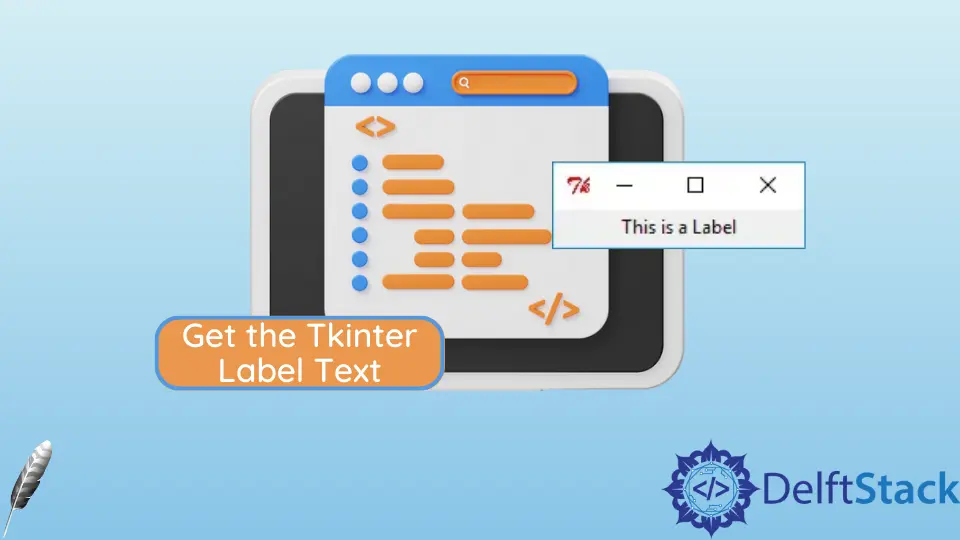
In this tutorial, we will introduce how to get the Tkinter Label
text by clicking a button.
cget
Method to Get text
Option Value of Tkinter Label
Tkinter Label
widget doesn’t have a specific get
method to get the text in the label. It has a cget
method to return the value of the specified option.
labelObj.cget("text")
It returns the text
property/opion of the Label
object - labelObj
.
Complete Working Example of cget
Method
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("200x80")
self.label = tk.Label(self.root, text="Text to be read")
self.button = tk.Button(
self.root, text="Read Label Text", command=self.readLabelText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def readLabelText(self):
print(self.label.cget("text"))
app = Test()
Read Value of Key text
of Label Object Dictionary to Get Tkinter Label
Text
A label object is also a Python dictionary, so we could get its text by accessing the "text"
key.
Complete Working Example
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("200x80")
self.label = tk.Label(self.root, text="Text to be read")
self.button = tk.Button(
self.root, text="Read Label Text", command=self.readLabelText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def readLabelText(self):
print(self.label["text"])
app = Test()
Use StringVar
to Get the Tkinter Label
Text
StringVar
is one type of Tkinter constructor to create the Tkinter string variable.
After we associate the StringVar
variable to the Tkinter widgets, we could get the text of the label by reading the value of StringVar
variable.
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("200x80")
self.text = tk.StringVar()
self.text.set("Text to be read")
self.label = tk.Label(self.root, textvariable=self.text)
self.button = tk.Button(
self.root, text="Read Label Text", command=self.readLabelText
)
self.button.pack()
self.label.pack()
self.root.mainloop()
def readLabelText(self):
print(self.text.get())
app = Test()
get()
method of StringVar
variable returns its value, which is associated with the label text in this example.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook