How to Freeze the Tkinter Window Frame Size
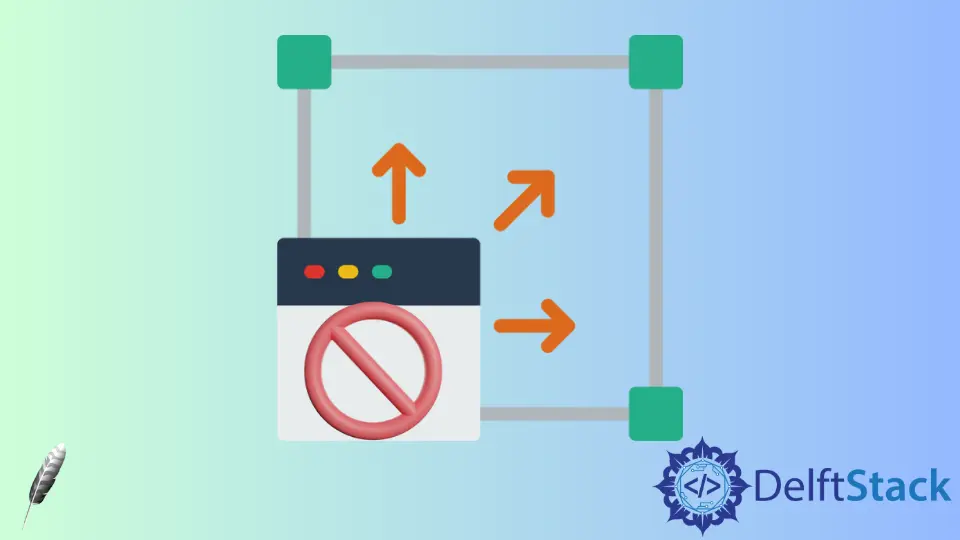
Creating a graphical user interface (GUI) with Python’s Tkinter library is both fun and rewarding. However, there are times when you may want to restrict the size of your Tkinter window. Freezing the window frame size can enhance user experience by preventing accidental resizing that might disrupt the layout.
In this tutorial, we’ll explore simple methods to achieve this, ensuring your application maintains a consistent look and feel. Whether you’re a beginner or an experienced developer, you’ll find these techniques straightforward and easy to implement. Let’s dive into the world of Tkinter and learn how to freeze the window frame size effectively.
Method 1: Using the resizable()
Method
One of the simplest ways to prevent a Tkinter window from being resized is by using the built-in resizable()
method. This method allows you to specify whether the window can be resized in the horizontal and vertical directions. By setting both parameters to False
, you effectively lock the window size.
Here’s how you can do it:
import tkinter as tk
root = tk.Tk()
root.title("Fixed Size Window")
root.geometry("400x300")
root.resizable(False, False)
label = tk.Label(root, text="This window cannot be resized.")
label.pack(pady=20)
root.mainloop()
Output:
In this example, we start by importing the Tkinter library and creating a main window. We set the title and size of the window using the geometry()
method. The crucial part comes with the resizable()
method, where both arguments are set to False
. This action locks the window size, preventing any resizing by the user. The label in the window provides a clear message indicating that the window is fixed in size. This method is straightforward and effective for most applications.
Method 2: Using the wm_attributes()
Method
Another approach to freeze the Tkinter window frame size is by utilizing the wm_attributes()
method. This method allows for more advanced window management, including disabling resizing features. By setting the -toolwindow
attribute, you can create a window that cannot be resized or minimized.
Here’s an example:
import tkinter as tk
root = tk.Tk()
root.title("Tool Window")
root.geometry("400x300")
root.wm_attributes("-toolwindow", True)
label = tk.Label(root, text="This is a tool window and cannot be resized.")
label.pack(pady=20)
root.mainloop()
Output:
In this code snippet, we again create a Tkinter window and set its title and size. The key difference here is the use of wm_attributes("-toolwindow", True)
, which modifies the window’s attributes to behave like a tool window. This effectively locks the window size, and users won’t be able to resize or minimize it. This method is particularly useful when creating utility applications where a fixed window size is essential.
Conclusion
Freezing the Tkinter window frame size is a straightforward task that can significantly enhance the user experience of your application. Whether you choose to use the resizable()
method for simplicity, the wm_attributes()
method for advanced control, each approach has its unique advantages. By implementing these techniques, you can create a professional-looking GUI that maintains its intended layout and functionality. Now that you have the knowledge, it’s time to put it into practice and create your own fixed-size Tkinter applications!
FAQ
-
how can I make a Tkinter window resizable again?
You can make a Tkinter window resizable by calling theresizable()
method again and setting its parameters toTrue
. -
can I set a minimum size for a Tkinter window?
Yes, you can set a minimum size for a Tkinter window usingminsize(width, height)
method. -
does freezing the window size affect the layout of widgets?
Freezing the window size does not affect the layout of widgets; however, it prevents users from changing the window dimensions. -
is it possible to lock only one dimension of the Tkinter window?
Yes, you can lock only one dimension by setting one parameter of theresizable()
method toFalse
and the other toTrue
. -
can I create a non-resizable window with a title bar?
Yes, using theresizable()
method will allow you to create a non-resizable window while still retaining the title bar.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook