How to Create a New Window by Clicking a Button in Tkinter
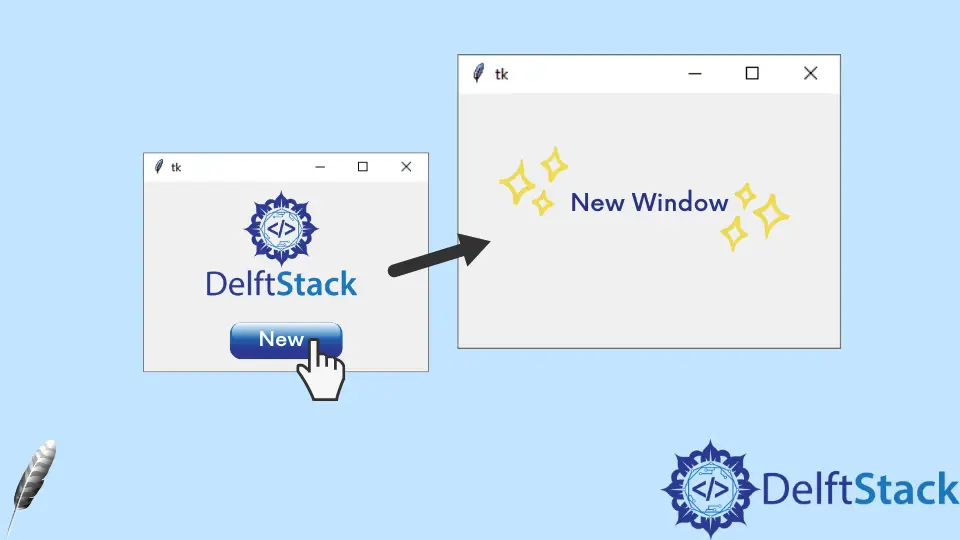
In this tutorial, we will show you how to create and open a new Tkinter window by clicking a button in Tkinter.
Create a New Tkinter Window
import tkinter as tk
def createNewWindow():
newWindow = tk.Toplevel(app)
app = tk.Tk()
buttonExample = tk.Button(app, text="Create new window", command=createNewWindow)
buttonExample.pack()
app.mainloop()
We normally use tk.Tk()
to create a new Tkinter window, but it is not valid if we have already created a root window as shown in the above codes.
Toplevel
is the right widget in this circumstance as the Toplevel
widget is intended to display extra pop-up
windows.
buttonExample = tk.Button(app, text="Create new window", command=createNewWindow)
It binds the createNewWindow
function to the button.
The new window is an empty window in the above example and you could add more widgets to it just like adding widgets in a normal root window, but need to change the parent widget to the created Toplevel
window.
import tkinter as tk
def createNewWindow():
newWindow = tk.Toplevel(app)
labelExample = tk.Label(newWindow, text="New Window")
buttonExample = tk.Button(newWindow, text="New Window button")
labelExample.pack()
buttonExample.pack()
app = tk.Tk()
buttonExample = tk.Button(app, text="Create new window", command=createNewWindow)
buttonExample.pack()
app.mainloop()
As you could see, labelExample
and buttonExample
have their parent widget as newWindow
but not app
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook