How to Delete Tkinter Text Box's Contents
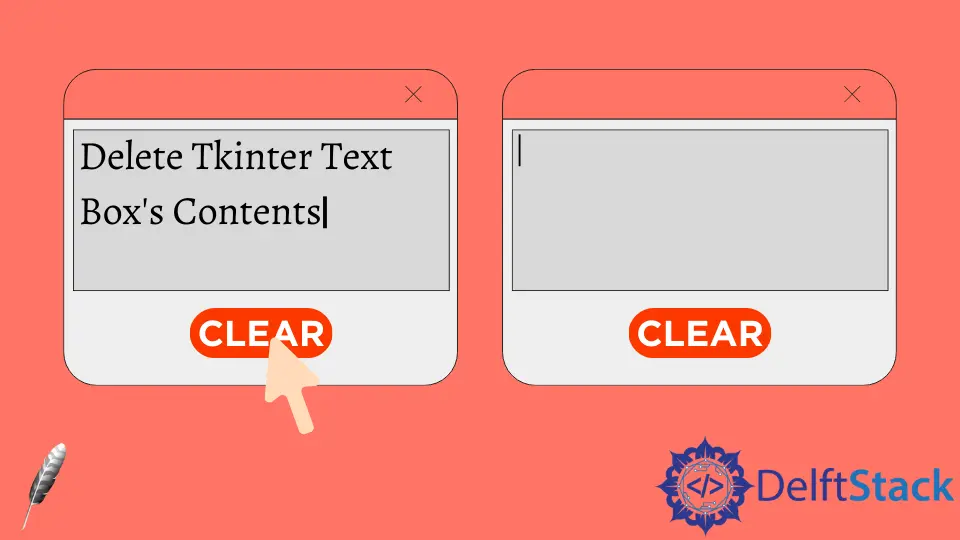
Tkinter Text widget has delete(first, last=None)
method to delete the characters at the index of first
, or within the range of (first, last)
from the text box.
If last
is not given, only the character specified in the first
position is deleted.
Example Code to Clear the Content of Tkinter Text Widget
import tkinter as tk
root = tk.Tk()
root.geometry("400x240")
def clearTextInput():
textExample.delete("1.0", "end")
textExample = tk.Text(root, height=10)
textExample.pack()
btnRead = tk.Button(root, height=1, width=10, text="Clear", command=clearTextInput)
btnRead.pack()
root.mainloop()
textExample.get("1.0", "end")
"1.0"
and "end"
refer to the first character and the last character of the contents in the Text
widget, the same as introduced in the article of how to get the input of the Text
widget.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook