How to Change Tkinter Button State
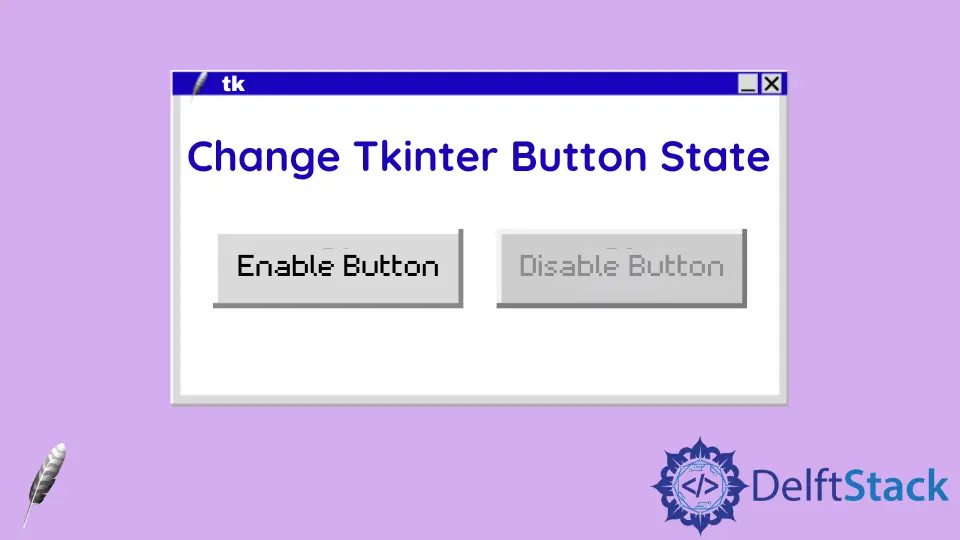
Tkinter Button has two states,
NORMAL
- The button could be clicked by the userDISABLE
- The button is not clickable
try:
import Tkinter as tk
except:
import tkinter as tk
app = tk.Tk()
app.geometry("300x100")
button1 = tk.Button(app, text="Button 1", state=tk.DISABLED)
button2 = tk.Button(app, text="EN/DISABLE Button 1")
button1.pack(side=tk.LEFT)
button2.pack(side=tk.RIGHT)
app.mainloop()
The left button is disabled (gray out) and the right button is normal.
The states could be modified in the dictionary-like method or configuration-like method.
try:
import Tkinter as tk
except:
import tkinter as tk
def switchButtonState():
if button1["state"] == tk.NORMAL:
button1["state"] = tk.DISABLED
else:
button1["state"] = tk.NORMAL
app = tk.Tk()
app.geometry("300x100")
button1 = tk.Button(app, text="Python Button 1", state=tk.DISABLED)
button2 = tk.Button(app, text="EN/DISABLE Button 1", command=switchButtonState)
button1.pack(side=tk.LEFT)
button2.pack(side=tk.RIGHT)
app.mainloop()
By clicking button2
, it calls the function switchButtonState
to switch button1
state from DISABLED
to NORMAL
, or vice versa.
state
is the option of Tkinter Button
widget. All the options in the Button
widget are the keys
of Button
as a dictionary.
def switchButtonState():
if button1["state"] == tk.NORMAL:
button1["state"] = tk.DISABLED
else:
button1["state"] = tk.NORMAL
The state
is updated by changing the value of state
in the Button
dictionary.
The state
could also be modified by using config
method of Button
object. Therefore, the switchButtonState()
function could also be implemented as below,
def switchButtonState():
if button1["state"] == tk.NORMAL:
button1.config(state=tk.DISABLED)
else:
button1.config(state=tk.NORMAL)
Even the strings normal
and disabled
could be simply used rather than tk.NORMAL
and tk.DISABLED
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook