How to Display Text in Pygame
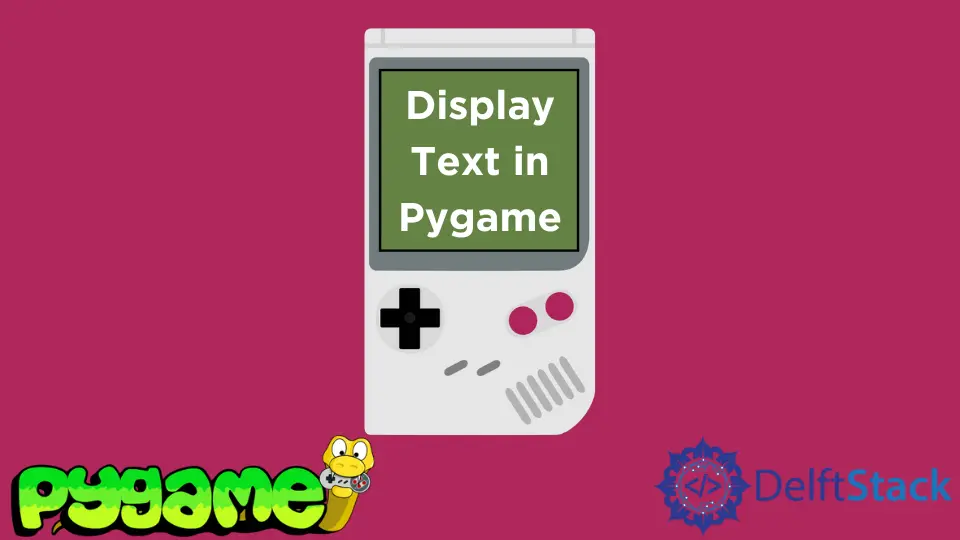
This tutorial teaches you how to draw text in Pygame.
The code displayed here is not the full code for a valid Pygame window. If you are interested in a bare-bones framework, consult this article.
Import the Font in Pygame
Before we can write something, we need to import a font. We can do this in two ways: either we use pygame.font.SysFont(fontname, size)
and we supply the font name as shown in the fonts folder on windows, or we use pygame.font.Font(font_file_path, size)
where we need to supply the path of a font file.
We first call the font
function in the example below but then overwrite the font
object with a SysFont
object. The first function won’t work for you unless you have a rocks
font file in your current working directory.
# or Font File in Directory
font = pygame.font.Font("rocks.ttf", 30)
# System Font
font = pygame.font.SysFont("Garamond", 30)
Display the Text in Pygame
After we have imported our font, we use the render()
method on it, and here, we can supply some interesting stuff.
- First, we pass the text that will be written.
- Then, we tell it whether the text should be antialiased.
- Lastly, we set the text color in an RGB fashion. Keep in mind that everything we did was before the main loop.
textsurface = font.render("Some Text", False, (200, 200, 200))
Now in the main game loop, we can call the blit
function on the screen object and then pass the textsurface
we just made with the desired position.
# keep in mind this code is in the main loop.
screen.blit(textsurface, (100, 100))
Complete Example Code
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height), pygame.RESIZABLE)
# or Font File in Directory
font = pygame.font.Font("rocks.ttf", 30)
# System Font
font = pygame.font.SysFont("Garamond", 30)
textsurface = font.render("Some Text", False, (200, 200, 200))
# Game loop.
while True:
screen.fill((20, 20, 20))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.blit(textsurface, (100, 100))
pygame.display.flip()
fpsClock.tick(fps)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub