pygame.display.set_mode in Pygame
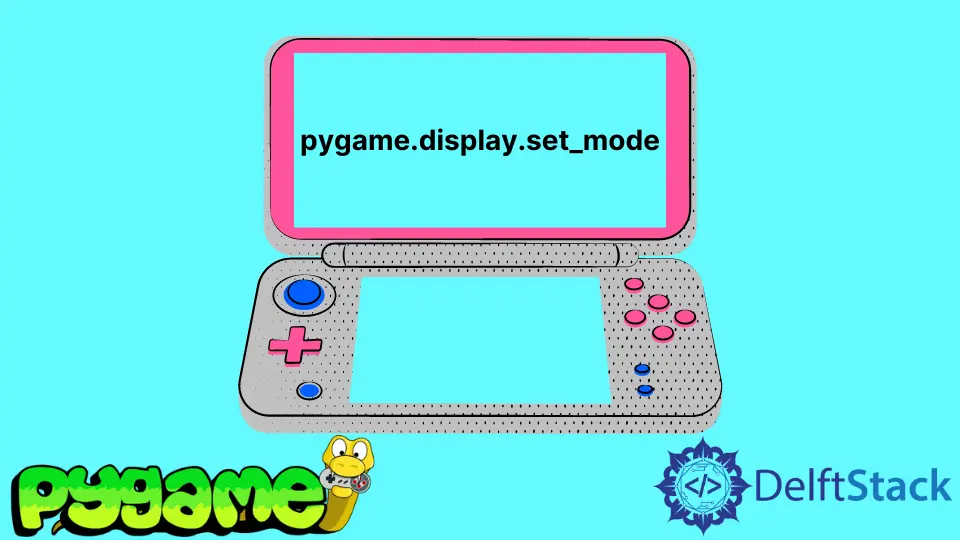
This article teaches you what the pygame.display.set_mode
function does in Pygame and how to correctly use it. The code displayed here is not the full code for a valid Pygame window.
If you are interested in a working framework, consult this article.
What Does the pygame.display.set_mode
Do in Pygame
In Pygame, most of the things we see when we run the games are so-called Surfaces
. These Surfaces
can be overlayed onto each other with the blit
function.
This means there is some layering when building your game. Now one layer has to be the bottom layer that is drawn nonetheless.
The pygame.display.set_mode
does help us achieve that. We can pass two arguments: the starting dimensions of the window and the window flags; we get to these later.
Most importantly, it will return a Surface
, often called a screen
. This Surface
is the Bottom Layer.
In the main loop, we blit everything onto this surface. We also need to call the pygame.display.flip
method, so our screen is updated.
Now to the flags of the pygame.display.set_mode
function. These determine how the window itself behaves, and the two most likely to use are pygame.FULLSCREEN
and pygame.RESIZEABLE
.
As their names suggest, they either make the window fill the whole screen or, in the case of pygame.RESIZEABLE
, allow the user to resize the window. The latter option requires some work to make your game responsive to the window size.
Correct Usage of the pygame.display.set_mode
The correct usage is pretty simple. Start before the main loop by calling the function and saving its return value to a variable, commonly called screen
.
screen = pygame.display.set_mode([width, height])
After that, we go into the main loop and call pygame.display.set_mode
at the end of the iteration.
Now you know what the pygame.display.set_mode
function does and how to correctly use it.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub