How to Draw a Circle in Pygame
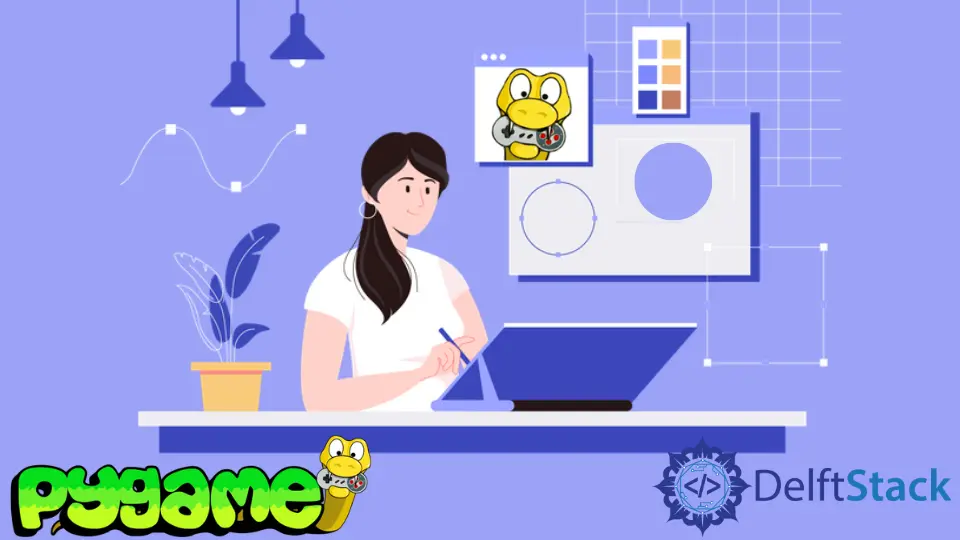
This tutorial teaches you how to draw circles in Pygame.
The code displayed here is not the complete code for a valid Pygame window. If you are interested in a bare-bones framework, consult this article.
Draw a Circle in Pygame
To draw a circle, we only need the pygame.draw.circle
method, which will provide the following arguments: Surface
, Color
, Center
, Radius
. The first one is just the surface we want to draw it on.
We draw it on the screen in our example, but we can use any surface. Then, we define the color in an RGB fashion with an array.
We continue by defining the circle center position on the previously mentioned surface. Last but not least, we define the radius.
Keep in mind that this code runs in the main loop of our game.
Code snippet:
pygame.draw.circle(
screen, # Surface to draw on
[100, 100, 100], # Color in RGB Fashion
[100, 100], # Center
20, # Radius
)
Output:
Draw a Half-Circle in Pygame
Maybe you want to make a half-circle. We can also do this with the pygame.draw.circle
method.
We just need to provide more arguments, which are: draw_top_right
, draw_top_left
, draw_bottom_left
, draw_bottom_right
. As their name suggests, they define whether this quarter will be drawn or not.
That’s why we work with booleans here. When we define one of them as true
, the others won’t be drawn, but we can define multiple corners simultaneously.
The following code will draw the upper part of the circle.
Code snippet:
pygame.draw.circle(
screen, # Surface to draw on
[100, 100, 100], # Color in RGB Fashion
[100, 100], # Center
20, # Radius
draw_top_right=True,
draw_top_left=True,
)
Output:
Complete Example Code
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height))
# Game loop.
while True:
screen.fill((20, 20, 20))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.draw.circle(
screen, # Surface to draw on
[100, 100, 100], # Color in RGB Fashion
[100, 100], # Center
20, # Radius
draw_top_right=True,
draw_top_left=True,
)
pygame.display.flip()
fpsClock.tick(fps)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub