How to Detect Collisions in Pygame
-
Detect Collisions Using
rect.collidepoint()
in Pygame -
Detect Collisions Using
rect.colliderect()
in Pygame -
Detect Collisions Using
rect.collidelist()
orrect.collidelistall()
in Pygame -
Detect Collisions Using
rect.collidedict()
orrect.collidedictall()
in Pygame
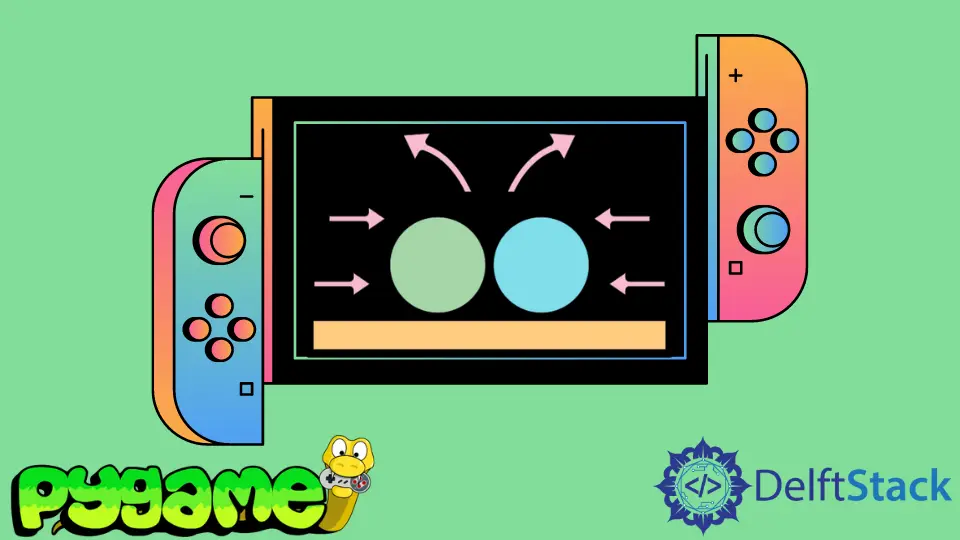
There are several different ways of detecting collisions in Pygame, and this article will cover some of them.
The code displayed here is not the complete code for a valid Pygame window. If you are interested in a bare-bones framework, consult this article.
Detect Collisions Using rect.collidepoint()
in Pygame
With the collidepoint()
method of the rect
class, we can test if a given point is inside the rect
. This is useful on many occasions, for example, when you want some UI elements to change the look when hovering over them.
In this article, we have discussed the function and made a button with it. You can either supply the function with the x
and y
coordinate separately or provide them in an iterable like a tuple or list.
Syntax:
rect.collidepoint(x, y)
rect.collidepoint((x, y))
Detect Collisions Using rect.colliderect()
in Pygame
With this function of the rect
class, we can test whether any portion of another rect
is within its bounds. Hence, we have to supply this function with another rect
.
Syntax:
rect.colliderect(rect)
Detect Collisions Using rect.collidelist()
or rect.collidelistall()
in Pygame
We can also use the rect.collidelist()
method, which receives a list of rects
and tests if one intersects with the main rect
. This function will return the first rect
index, which collides with the rect
.
The rect.collidelistall()
method is similar and has only one key difference: it will return an array with all indices of rects
that collide with the main rect
.
Syntax:
rect.collidelist([rect1, rect2, ...])
rect.collidelistall([rect1, rect2, ...])
Detect Collisions Using rect.collidedict()
or rect.collidedictall()
in Pygame
These methods are pretty much the same as the methods above, but they return the key and value pair of rects
that collide with the main rect
.
Syntax:
rect.collidedict({
'rect1': rect1,
'rect2': rect2,
...
})
rect.collidedictall({
'rect1': rect1,
'rect2': rect2,
...
})
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub