KEYDOWN Function in Pygame
- Understanding Pygame and Event Handling
- Basic Implementation of the KEYDOWN Function
- Advanced Usage: Key Combinations and Actions
- Implementing Multiple Key Presses
- Conclusion
- FAQ
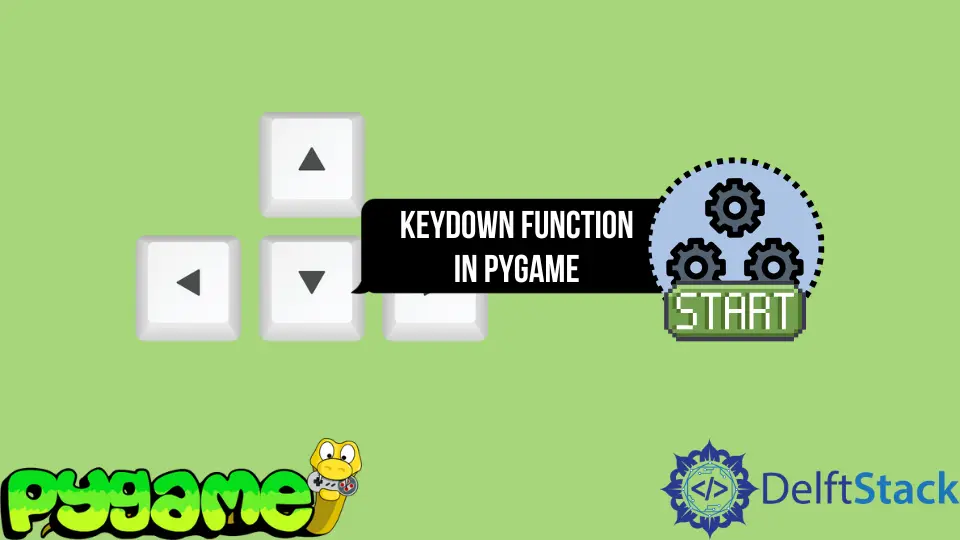
Pygame is a popular library in Python that simplifies game development. One of its essential features is the KEYDOWN function, which detects when a key is pressed down. This function is crucial for creating interactive experiences in games, allowing developers to implement controls, character movements, and various gameplay mechanics.
In this tutorial, we will explore how to effectively use the KEYDOWN function in Pygame, providing you with practical examples and clear explanations. Whether you are a beginner or an experienced developer, understanding the KEYDOWN function can significantly enhance your game design skills. Let’s dive into the world of Pygame and discover how this function can bring your game to life!
Understanding Pygame and Event Handling
Before we dive into the specifics of the KEYDOWN function, it’s essential to understand how Pygame handles events. Pygame operates on an event loop, which continuously checks for user inputs, such as keyboard presses or mouse movements. When a user interacts with the game, Pygame generates events that your code can respond to. The KEYDOWN event is triggered whenever a key is pressed down, allowing you to execute specific actions in your game.
To get started with Pygame, ensure you have it installed. You can do this using pip:
pip install pygame
Once Pygame is installed, you can begin coding your game. The following sections will illustrate how to use the KEYDOWN function effectively.
Basic Implementation of the KEYDOWN Function
To demonstrate the KEYDOWN function, we’ll create a simple Pygame window that responds to key presses. The following code sets up a basic window and listens for key presses to change the background color.
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((600, 400))
pygame.display.set_caption('KEYDOWN Example')
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_r:
screen.fill((255, 0, 0)) # Red
elif event.key == pygame.K_g:
screen.fill((0, 255, 0)) # Green
elif event.key == pygame.K_b:
screen.fill((0, 0, 255)) # Blue
pygame.display.flip()
Output:
A window opens, and pressing R, G, or B changes the background color.
In this code, we initialize Pygame and create a window. The event loop checks for events, specifically the KEYDOWN event. Depending on the key pressed (R, G, or B), the background color of the window changes accordingly. This simple example illustrates how the KEYDOWN function can be utilized for user interaction in Pygame.
Advanced Usage: Key Combinations and Actions
While the basic implementation of the KEYDOWN function is useful, you can enhance your game by responding to key combinations or executing specific actions. Let’s expand our previous example to include more complex interactions, such as moving a character on the screen.
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((600, 400))
pygame.display.set_caption('KEYDOWN Advanced Example')
x, y = 300, 200
speed = 5
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
x -= speed
if keys[pygame.K_RIGHT]:
x += speed
if keys[pygame.K_UP]:
y -= speed
if keys[pygame.K_DOWN]:
y += speed
screen.fill((0, 0, 0)) # Clear screen
pygame.draw.rect(screen, (255, 255, 255), (x, y, 50, 50)) # Draw character
pygame.display.flip()
Output:
A white square moves around the screen when arrow keys are pressed.
In this code, we first initialize the Pygame window and set the starting position of a square (representing a character). Instead of checking for the KEYDOWN event, we use pygame.key.get_pressed()
to continuously check the state of the keyboard. This allows for smooth movement of the square when the arrow keys are pressed. By adjusting the position of the square based on key inputs, we create a more interactive experience for the player.
Implementing Multiple Key Presses
One of the powerful features of the KEYDOWN function is the ability to handle multiple key presses simultaneously. This is particularly useful in games where you may want to allow for diagonal movement or complex actions triggered by combinations of keys. Let’s modify our previous example to enable diagonal movement.
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((600, 400))
pygame.display.set_caption('KEYDOWN Multiple Keys Example')
x, y = 300, 200
speed = 5
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
x -= speed
if keys[pygame.K_RIGHT]:
x += speed
if keys[pygame.K_UP]:
y -= speed
if keys[pygame.K_DOWN]:
y += speed
screen.fill((0, 0, 0))
pygame.draw.rect(screen, (255, 255, 255), (x, y, 50, 50))
pygame.display.flip()
Output:
The square can move diagonally when two arrow keys are pressed simultaneously.
In this example, the square can move in any direction based on the arrow keys pressed. By utilizing pygame.key.get_pressed()
, we can check the state of multiple keys at once, allowing for diagonal movement. This feature enhances gameplay by providing players with more control over their characters.
Conclusion
In this tutorial, we explored the KEYDOWN function in Pygame, starting from its basic implementation to more advanced techniques that allow for complex interactions. Understanding how to utilize the KEYDOWN function effectively can significantly improve your game development skills, enabling you to create engaging and interactive experiences. As you continue to develop your games, consider experimenting with different key events and combinations to see how they can enhance your gameplay. Happy coding!
FAQ
-
What is the KEYDOWN function in Pygame?
The KEYDOWN function in Pygame detects when a key is pressed down, allowing developers to implement interactive controls in their games. -
How do I install Pygame?
You can install Pygame using pip by running the commandpip install pygame
in your terminal. -
Can I use the KEYDOWN function for multiple keys?
Yes, by usingpygame.key.get_pressed()
, you can check the state of multiple keys simultaneously for actions like diagonal movement. -
Is Pygame suitable for beginners?
Absolutely! Pygame is user-friendly and provides a great platform for beginners to learn game development in Python. -
What kind of games can I create with Pygame?
You can create a wide range of games with Pygame, from simple 2D platformers to more complex arcade-style games.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub