How to Get Available Fonts in Pygame
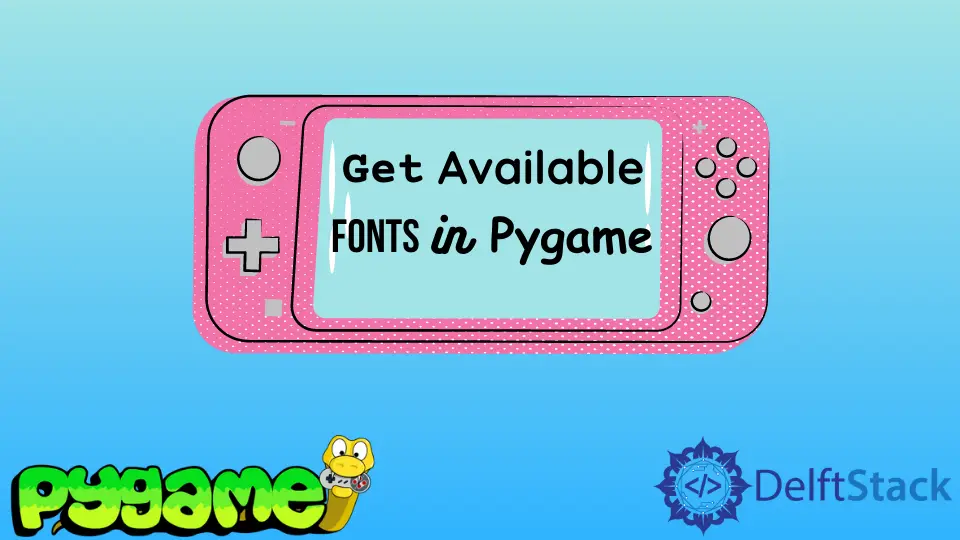
This short tutorial teaches you to get all available fonts and import them into Pygame.
Get Available Fonts With pygame.font.get_fonts()
To get all available system fonts, call the pygame.font.get_fonts()
method of Pygame. This will return an array containing lowercase font names.
This is useful when working with pygame.font.SysFont()
, which wants the font name as the first Argument.
Code:
print(pygame.font.get_fonts())
The output could look like this. Of course, this depends on the fonts installed on your device.
Output:
['arial', 'arialblack', 'bahnschrift', ...]
Difference Between SysFont
and Font
There are two ways to import fonts in Pygame. Through pygame.font.SysFont
and pygame.font.Font
.
The difference is that the SysFont
class expects a font name, and the Font
class expects a path to a font file, for example, a .ttf
font. The get_fonts()
function is needed for SysFont
because it returns the font names.
Complete Code:
# Imports
import sys
import pygame
# Configuration
pygame.init()
print(pygame.font.get_fonts())
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub