How to Draw Rectangle in Pygame
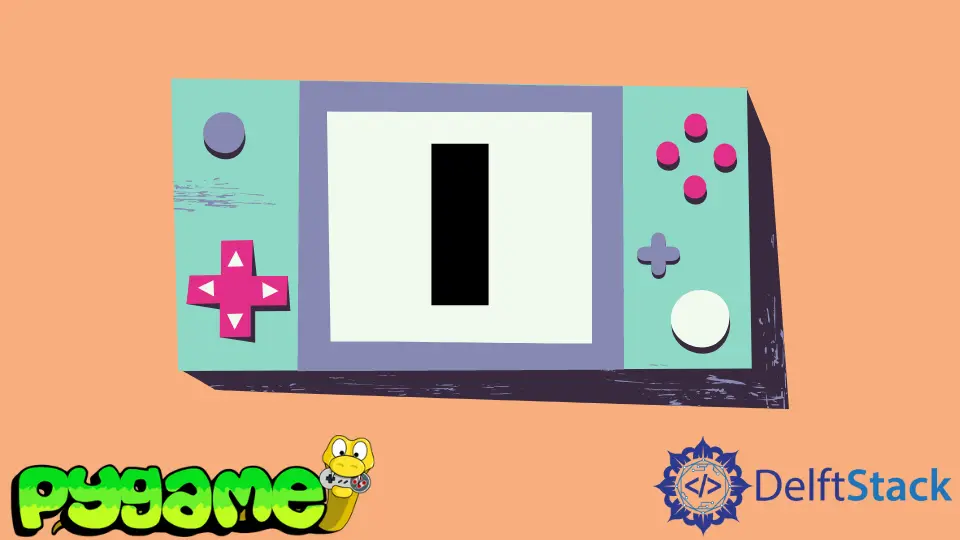
This tutorial will discuss how to draw a rectangle in Pygame.
The code displayed here is not the full code for a valid Pygame window. If you are interested in a bare-bones framework, consult this article.
Draw Rectangle in Pygame
We need one function to draw a rectangle: pygame.draw.rect()
. We call it inside the main loop, and we need the following arguments for it to work correctly: surface, color, position, and extent.
The first one is simply the screen we defined earlier; then, we continue with the color here in the RGB fashion. Then we initialize an array with four values.
The first two are the position, and the last two are the width and height of the drawn rectangle.
pygame.draw.rect(
screen,
[200, 200, 200], # Color in RGB Fashion
[100, 100, 30, 60], # left, top, width, height
)
Output:
Complete Example Code
# Imports
import sys
import pygame
# Configuration
pygame.init()
fps = 60
fpsClock = pygame.time.Clock()
width, height = 640, 480
screen = pygame.display.set_mode((width, height), pygame.RESIZABLE)
# Game loop.
while True:
screen.fill((20, 20, 20))
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
pygame.draw.rect(screen, [200, 200, 200], [100, 100, 30, 60], border_radius=100)
pygame.display.flip()
fpsClock.tick(fps)
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub