Paramiko Python
- What is Paramiko?
- Installing Paramiko
- Connecting to a Remote Server
- Executing Commands Remotely
- Transferring Files Using SFTP
- Conclusion
- FAQ
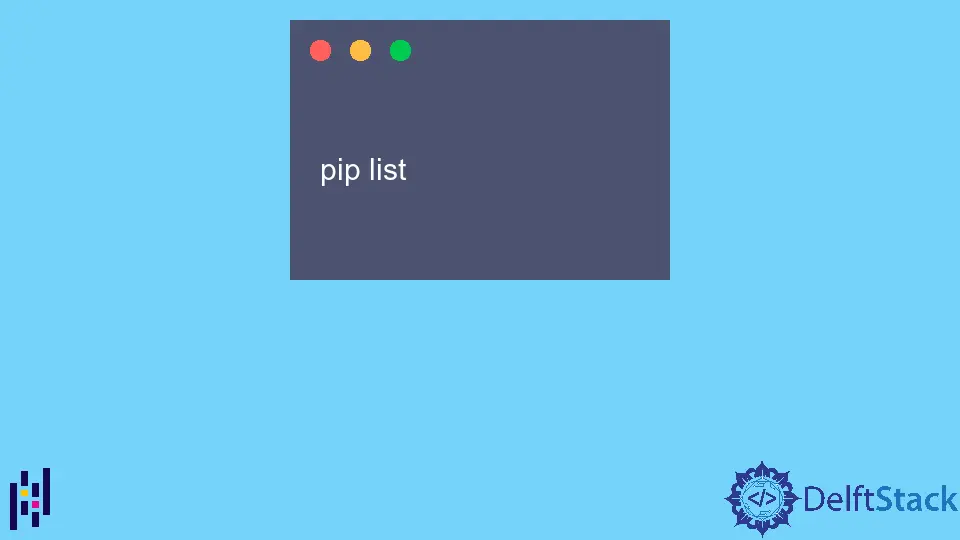
In the world of Python programming, Paramiko stands out as a powerful library for handling SSH connections. Whether you want to automate server management tasks, transfer files securely, or execute commands remotely, Paramiko provides a robust solution.
This tutorial will walk you through what Paramiko is, how to install it, and how to use it effectively in your Python projects. By the end of this guide, you’ll have a solid understanding of how to leverage Paramiko to enhance your applications. Let’s dive in!
What is Paramiko?
Paramiko is a Python implementation of the SSH (Secure Shell) protocol. It allows users to connect to remote servers securely, execute commands, and transfer files over the network. This library is particularly useful for system administrators and developers who need to manage remote systems programmatically. Paramiko supports both SSH1 and SSH2 protocols and is built on top of the cryptography library, ensuring secure connections. With features like key-based authentication, secure file transfer, and the ability to run commands remotely, Paramiko is a versatile tool in any Python developer’s toolkit.
Installing Paramiko
Before you can start using Paramiko, you need to install it. The installation process is straightforward and can be done using pip, Python’s package manager. Here’s how you can install Paramiko on your system.
pip install paramiko
After running this command in your terminal or command prompt, you should see a message indicating that Paramiko has been successfully installed.
Output:
Successfully installed paramiko-2.7.2
Once installed, you can verify the installation by importing Paramiko in a Python shell or script. If there are no errors, you’re ready to start using it.
import paramiko
If you encounter any issues during installation, ensure that your Python environment is correctly set up and that you have the necessary permissions to install packages.
Connecting to a Remote Server
Now that you have Paramiko installed, let’s explore how to establish a connection to a remote server. This is the foundation for using Paramiko effectively. Here’s a simple example that demonstrates how to connect using SSH.
import paramiko
hostname = "your_remote_server.com"
username = "your_username"
password = "your_password"
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, username=username, password=password)
In this code, we first import the Paramiko library. We then define the hostname, username, and password for the remote server. The SSHClient()
method creates a new SSH client instance, and set_missing_host_key_policy()
is used to automatically add the server’s host key if it’s not already known. Finally, the connect()
method establishes the connection.
Output:
Connection established to your_remote_server.com
After executing this code, you should see a message confirming that the connection has been established. It’s crucial to handle exceptions here, as network issues or incorrect credentials can lead to connection failures. You can use try-except blocks to manage these scenarios gracefully.
Executing Commands Remotely
Once you have a successful connection, you can execute commands on the remote server. This is particularly useful for automating tasks. Here’s how you can run a command and retrieve its output.
stdin, stdout, stderr = client.exec_command("ls -l")
output = stdout.read().decode()
print(output)
In this snippet, we use the exec_command()
method to run the command ls -l
, which lists files in the current directory in long format. The method returns three file-like objects: stdin
, stdout
, and stderr
. We read from stdout
to get the command’s output and decode it to convert it from bytes to a string.
Output:
total 64
drwxr-xr-x 2 user group 4096 Oct 21 10:00 dir1
-rw-r--r-- 1 user group 123 Oct 21 10:00 file1.txt
This command provides you with a list of files and directories in the current working directory of the remote server. You can replace "ls -l"
with any command you wish to execute. Remember to handle errors by checking stderr
for any issues that may arise during command execution.
Transferring Files Using SFTP
Paramiko also allows you to transfer files securely between your local machine and a remote server using SFTP (SSH File Transfer Protocol). Here’s how you can upload a file.
sftp = client.open_sftp()
sftp.put("local_file.txt", "/remote_path/remote_file.txt")
sftp.close()
In this example, we first open an SFTP session using open_sftp()
. The put()
method uploads a local file to the specified remote path. After the upload is complete, we close the SFTP session.
Output:
File uploaded successfully
This simple process allows you to transfer files securely without needing additional tools. You can also use the get()
method to download files from the remote server to your local machine. Always ensure that you have the necessary permissions for the directories you are accessing.
Conclusion
Paramiko is a powerful and versatile library that simplifies SSH connections and remote server management in Python. From connecting to remote servers and executing commands to securely transferring files, Paramiko offers a comprehensive set of features for developers and system administrators alike. By following the steps outlined in this tutorial, you can harness the full potential of Paramiko in your projects. Start automating your server management tasks today and experience the benefits of using Python for remote operations!
FAQ
-
what is paramiko used for?
Paramiko is used for handling SSH connections, executing commands remotely, and transferring files securely in Python. -
how do I install paramiko?
You can install Paramiko using pip by running the commandpip install paramiko
in your terminal. -
can I use paramiko for file transfers?
Yes, Paramiko supports SFTP, allowing you to transfer files securely between your local machine and remote servers. -
what is the difference between exec_command and open_sftp?
exec_command
is used to execute shell commands on the remote server, whileopen_sftp
is used to open an SFTP session for file transfers. -
is paramiko secure?
Yes, Paramiko uses SSH, which is a secure protocol for remote connections and file transfers.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn