Pandas tz_localize
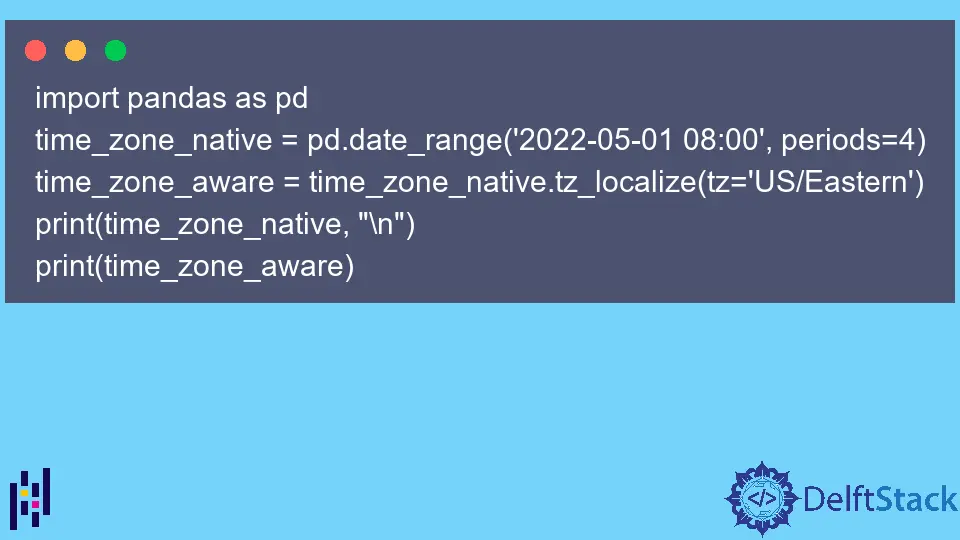
The Pandas library in Python makes performing data analysis-related functions super efficient with its wide variety of tools and methods. One such method is tz_localize
.
In this article, we will explore the functionality of the method.
the tz_localize()
Method in Python
The tz_localize()
method is used to localize the time zone’s native index of either a Series or a DataFrame, depending on the use case scenario, to a specifically targeted zone. This method makes the index localized.
Below is the straightforward syntax for the method.
DataFrame.tz_localize(
tz, axis=0, level=None, copy=True, ambiguous="raise", nonexistent="raise"
)
In the method above, each parameter is a value we can provide to the method to specify the time zone we want to localize more accurately.
tz
- We specify a valid time zone in this parameter.axis
- This is the axis we localize.level
- It is essentially the level of the datetime if there is more than one index.copy
- It is a Boolean value and carries out the function of copying any underlying data.ambiguous
- This parameter decides if the time zone is unclear because of DST.nonexistent
- It ensures that a timezone is defined if or when none can be found when the clocks move forward because of DST.
Now that we have covered the syntax and understood the basic functionality of the method let’s move on to a practical example script that utilizes this method.
import pandas as pd
time_zone_native = pd.date_range("2022-05-01 08:00", periods=4)
time_zone_aware = time_zone_native.tz_localize(tz="US/Eastern")
print(time_zone_native, "\n")
print(time_zone_aware)
Output:
As observed in the script above, we start by using the date_range()
method to define the exact date when we want our ’localizing’ to begin, along with the periods
parameter defining the next number of days that the method should extend to. We call this datetime index time_zone_native
.
In the case of our script, it is four days. Following that, we apply the tz_localize()
method to the datetime index we defined above. Then we print out the results.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn