How to Convert Pandas to CSV Without Index
- Method 1: Using the to_csv() Function
- Method 2: Specifying the Path and File Name
- Method 3: Using Different Delimiters
- Conclusion
- FAQ
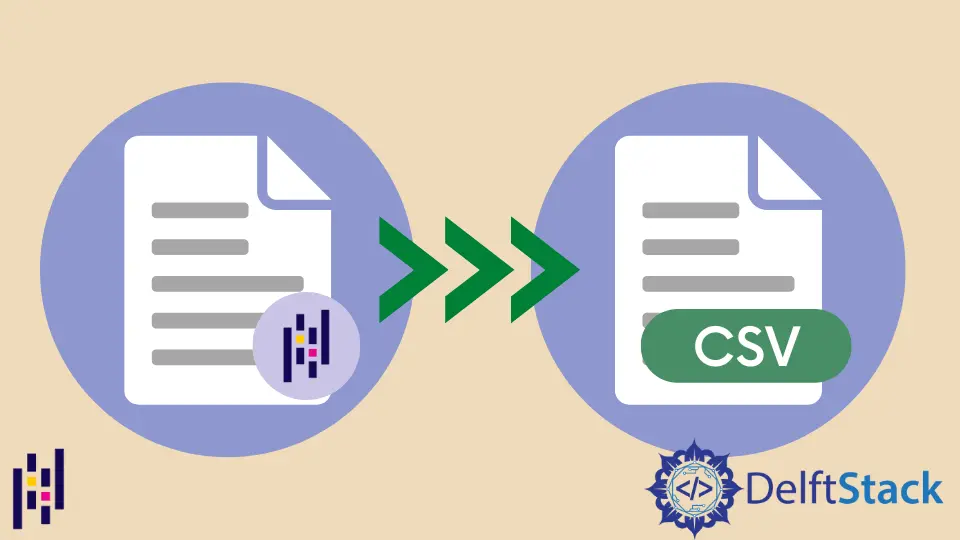
Converting a Pandas DataFrame to a CSV file is a common task in data analysis and manipulation. However, sometimes you may want to save your DataFrame without the index.
This tutorial will guide you through the process of converting a Pandas DataFrame to a CSV file while omitting the index. Whether you’re preparing data for export or simply want to clean up your CSV output, this guide will provide you with clear, step-by-step instructions. By the end, you’ll be equipped with the knowledge to easily manage your data export needs without the clutter of unnecessary index columns.
Method 1: Using the to_csv() Function
The most straightforward way to convert a Pandas DataFrame to a CSV file without including the index is by using the built-in to_csv()
function. This method is not only simple but also very efficient. Here’s how you can do it:
import pandas as pd
data = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']
}
df = pd.DataFrame(data)
df.to_csv('output.csv', index=False)
Output:
Name,Age,City
Alice,25,New York
Bob,30,Los Angeles
Charlie,35,Chicago
In this example, we first import the Pandas library and create a simple DataFrame containing names, ages, and cities. The to_csv()
function is then called on the DataFrame, specifying the filename output.csv
. The critical parameter here is index=False
, which tells Pandas to exclude the index from the output CSV file. This results in a clean CSV file that only contains the data you want, making it easier to read and analyze later.
Method 2: Specifying the Path and File Name
If you want to have more control over the file path or name when saving your CSV, you can specify these details directly in the to_csv()
function. This method is particularly useful when you are working with multiple data exports and need to organize your files efficiently.
import pandas as pd
data = {
'Product': ['Laptop', 'Tablet', 'Smartphone'],
'Price': [1000, 500, 700],
'Stock': [50, 150, 200]
}
df = pd.DataFrame(data)
df.to_csv('/path/to/your/directory/products.csv', index=False)
Output:
Product,Price,Stock
Laptop,1000,50
Tablet,500,150
Smartphone,700,200
In this example, we create another DataFrame representing products, their prices, and stock levels. By specifying the full path in the to_csv()
method, you can save your CSV to a designated directory. Again, the index=False
parameter ensures that the index is not included in the output. This method is especially beneficial for data organization, allowing you to keep your files structured and accessible.
Method 3: Using Different Delimiters
Sometimes, you may want to save your DataFrame using a different delimiter instead of the default comma. This can be useful for compatibility with other systems or for personal preference. The to_csv()
function allows you to specify a different delimiter easily.
import pandas as pd
data = {
'Employee': ['John', 'Doe', 'Jane'],
'Salary': [70000, 80000, 90000],
'Department': ['HR', 'IT', 'Finance']
}
df = pd.DataFrame(data)
df.to_csv('employees.tsv', sep='\t', index=False)
Output:
Employee Salary Department
John 70000 HR
Doe 80000 IT
Jane 90000 Finance
In this example, we create a DataFrame for employees, their salaries, and departments. By using the sep='\t'
parameter in the to_csv()
function, we save the DataFrame as a tab-separated values (TSV) file instead of a CSV. The index=False
parameter is still in play, ensuring that no index is included in the output. This flexibility allows you to adjust the format of your output according to your needs.
Conclusion
Converting a Pandas DataFrame to a CSV file without the index is a straightforward process that can greatly enhance the readability and usability of your data. Whether you’re using the basic to_csv()
function, specifying file paths, or changing delimiters, these methods make it easy to export your data cleanly. By applying these techniques, you can ensure that your exported data is organized, accessible, and ready for further analysis or sharing. With the right approach, managing your data exports can be both efficient and effective.
FAQ
-
How do I convert a DataFrame to CSV with the index?
You can convert a DataFrame to CSV with the index by using theto_csv()
function without theindex=False
parameter. By default, the index will be included. -
Can I specify a different file format when exporting?
Yes, you can specify different formats by changing the file extension in the filename, such as.tsv
for tab-separated values. -
What happens if I don’t specify a file path?
If you don’t specify a file path, the CSV file will be saved in the current working directory of your Python script. -
Is it possible to append data to an existing CSV file?
Yes, you can append data to an existing CSV file by using themode='a'
parameter in theto_csv()
function. -
Can I customize the column order in the output CSV?
Yes, you can customize the column order by selecting the columns in the desired order when calling theto_csv()
function.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum