How to Convert Timestamp to Datetime in Pandas
-
Use
Timestamp
as the Time of Events in Pandas -
the
Datetime
in Pandas -
Use
to_pydatetime()
Function to Convert Timestamp to Datetime in Pandas - Conclusion
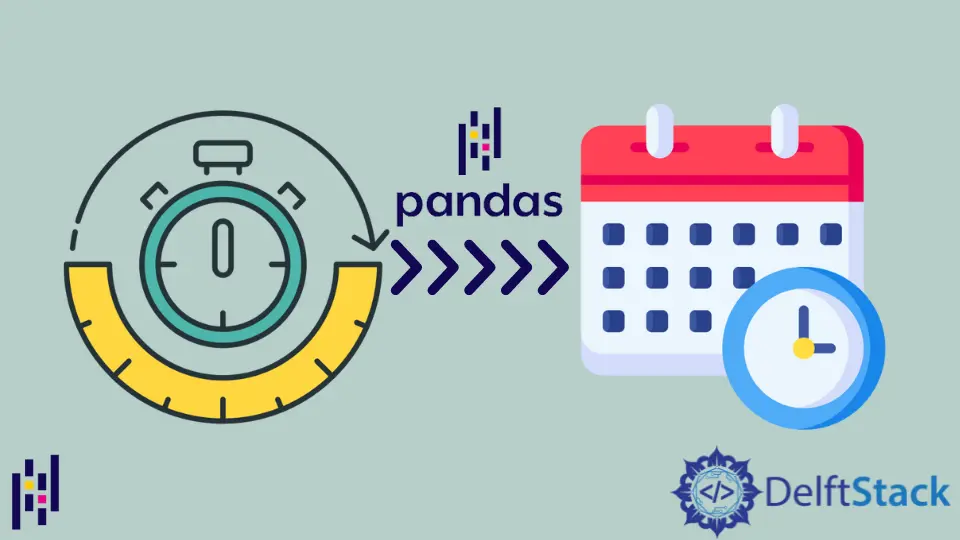
Pandas is an advanced data analysis
tool or a package extension in Python.
The timestamp
is the time of the event, while datetime
is used to analyze and manipulate data. This is why we must learn how to convert timestamps to datetime.
Use Timestamp
as the Time of Events in Pandas
First, we will create a dummy data frame to work with.
import pandas as pd
ts = pd.Timestamp("2014-01-23 00:00:00", tz=None)
The code block creates a data variable
with the value as specified for a specific timestamp
associated with an event.
print(ts)
Output:
2014-01-23 00:00:00
This timestamp represents the year, month, day, hour, minutes, and seconds exactly during the event.
This data is generally fetched from a local computer and is not analysis-friendly.
the Datetime
in Pandas
Datetime
is one of the most common data analysis types. It helps trace the movement and activity of a product.
It also helps in understanding the engagement of the product in the market.
Use to_pydatetime()
Function to Convert Timestamp to Datetime in Pandas
ts.to_pydatetime()
Output.
datetime.datetime(2014, 1, 23, 0, 0)
As we can see, the timestamp has been converted to a datetime
format.
Conclusion
Therefore, with the help of the to_pydatetime()
function in Pandas, we can efficiently manipulate our data to have our timestamp
converted to datetime
.