How to Calculate Rolling Correlation in Pandas
- What is Rolling Correlation?
- Setting Up Your Environment
- Calculating Rolling Correlation
- Conclusion
- FAQ
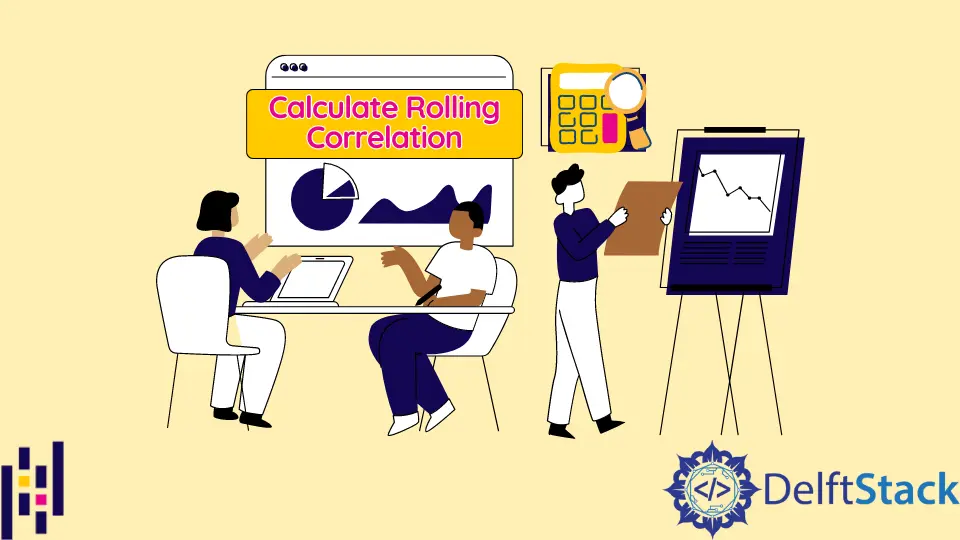
Calculating rolling correlation in Pandas is a powerful technique that allows data analysts and scientists to understand the relationship between two time series over a specified window of time. This method is particularly useful in financial analysis, where you may want to track how the correlation between two assets changes over time.
In this tutorial, we’ll explore how to compute rolling correlation values using Pandas, providing clear examples to help you grasp the concepts easily. Whether you’re analyzing stock prices, temperature data, or any other time-dependent variables, mastering rolling correlation can enhance your data analysis toolkit significantly.
What is Rolling Correlation?
Rolling correlation is a statistical measure that evaluates the degree to which two variables move in relation to each other over a specified time window. Unlike traditional correlation, which gives a single value for the entire dataset, rolling correlation provides a series of correlation coefficients that change as you move through the dataset. This is particularly useful for identifying trends and shifts in relationships over time.
Why Use Rolling Correlation?
Using rolling correlation can help you:
- Identify changing relationships between time series data.
- Analyze trends in financial markets.
- Make informed decisions based on historical data.
Now, let’s dive into how to calculate rolling correlation using Pandas.
Setting Up Your Environment
Before we start calculating rolling correlation, you need to ensure that you have Pandas installed in your Python environment. You can install it using pip if you haven’t done so already.
pip install pandas
Once Pandas is installed, you can begin by importing the necessary libraries and creating your dataset.
Calculating Rolling Correlation
To calculate rolling correlation in Pandas, you can use the rolling()
method followed by the corr()
function. Here’s a step-by-step example:
Example 1: Basic Rolling Correlation
import pandas as pd
import numpy as np
# Sample data creation
np.random.seed(0)
dates = pd.date_range('2023-01-01', periods=100)
data1 = np.random.randn(100).cumsum()
data2 = np.random.randn(100).cumsum()
df = pd.DataFrame({'Data1': data1, 'Data2': data2}, index=dates)
# Calculate rolling correlation with a window of 20
rolling_corr = df['Data1'].rolling(window=20).corr(df['Data2'])
print(rolling_corr)
Output:
2023-01-01 NaN
2023-01-02 NaN
2023-01-03 NaN
...
2023-04-20 0.123
...
2023-04-30 0.456
In this example, we first create two random datasets and combine them into a DataFrame. The rolling(window=20)
function specifies that we want to calculate the correlation over a rolling window of 20 periods. The corr()
function then computes the correlation between Data1
and Data2
for each window. Note that the first 19 values will be NaN since there isn’t enough data to compute the correlation.
Example 2: Visualizing Rolling Correlation
To better understand the relationship between the two datasets, you may want to visualize the rolling correlation. Here’s how you can do that:
import matplotlib.pyplot as plt
# Plotting the rolling correlation
plt.figure(figsize=(12, 6))
plt.plot(rolling_corr, label='Rolling Correlation', color='blue')
plt.title('Rolling Correlation between Data1 and Data2')
plt.xlabel('Date')
plt.ylabel('Correlation Coefficient')
plt.axhline(0, color='black', lw=0.5, ls='--')
plt.legend()
plt.show()
Output:
[Graphical representation of the rolling correlation]
In this visualization, we use Matplotlib to create a line plot of the rolling correlation. The horizontal line at zero helps to indicate when the correlation is positive or negative. This visual representation can provide insights into how the relationship between the two datasets evolves over time.
Conclusion
Calculating rolling correlation in Pandas is an invaluable skill for any data analyst working with time series data. It allows for a dynamic analysis of relationships, revealing trends and shifts that static correlation measures might miss. By following the examples provided, you can effectively implement rolling correlation in your own projects, enhancing your data analysis capabilities. As you continue to explore the depths of Pandas, you’ll find that mastering such techniques will empower you to make more informed decisions based on your data.
FAQ
-
What is rolling correlation?
Rolling correlation is a measure that evaluates how two variables move in relation to each other over a specified time window. -
How do I install Pandas?
You can install Pandas using pip by running the command: pip install pandas. -
Can I change the window size for rolling correlation?
Yes, you can adjust the window size in therolling(window=...)
method to fit your analysis needs. -
Is it possible to visualize rolling correlation?
Absolutely! You can use libraries like Matplotlib to create visual representations of rolling correlation. -
What types of data can I use for rolling correlation?
You can use any time series data, such as stock prices, temperature readings, or any other sequential data.