How to Read CSV Only Specific Columns Into Pandas DataFrame
-
Use the
read_csv()
Function and Pass a List of Column Names to Be Read -
Use the
read_csv()
Function and Pass List of Column Numbers to Be Read
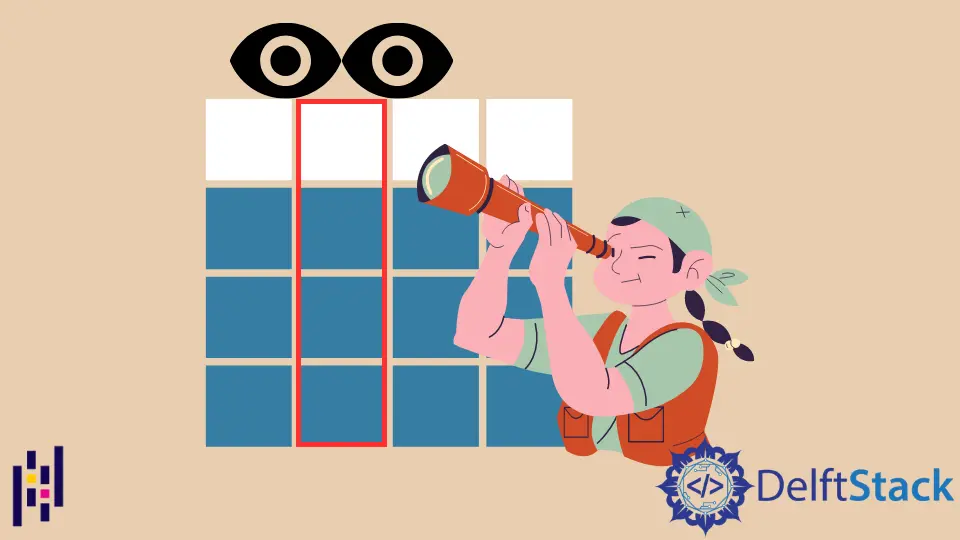
This tutorial will discuss different methods to read specific columns from a csv file using Pandas. We’ll use the following CSV file for the demo.
Course | Mentor | price $ |
|
---|---|---|---|
0 | Python | Robert | 199 |
1 | Spark | Elizibeth | 299 |
2 | Java | Nolan | 99 |
3 | JavaScript | Chris | 250 |
4 | C# | johnson | 399 |
Use the read_csv()
Function and Pass a List of Column Names to Be Read
The Pandas library provides read_csv()
function. The read_csv()
is used to read a comma-separated values (csv) file into DataFrame
.
The read_csv()
takes the file path of the csv file, and it has more than 40 optional parameters. For this tutorial, let’s concentrate usecols
parameter.
Syntax - pd.read_csv()
:
pd.read_csv(filepath, usecols)
Parameters
filepath
- The path or location of the csv file to be loaded.usecols
- List of specific columns to be read.
Returns
DataFrame
Follow the steps below to use the read_csv()
function and pass the list of column names to be read.
-
Import pandas library.
-
Pass the path of csv file and list of columns to be read to the
read_csv()
method. -
The
read_csv()
method will return a data frame with that specific columns. -
Print the
DataFrame
.
The following code is the implementation of the above approach.
# import the pandas
import pandas as pd
# Reading the specific columns from csv
df = pd.read_csv("data.csv", usecols=["Course", "price$"])
# printing the dataframe
print(df)
Output:
Course price$
0 Python 199
1 Spark 299
2 Java 99
3 JavaScript 250
4 C# 399
Use the read_csv()
Function and Pass List of Column Numbers to Be Read
We can also use the column numbers to read_csv()
to read-only specific columns.
-
Import pandas library.
-
Pass the path of csv file and list of columns numbers to be read to the
read_csv()
method. -
The
read_csv()
method will return a data frame with that specific columns. -
Print the
DataFrame
.
The following code is the implementation of the above approach.
# import the pandas
import pandas as pd
# Reading the specific columns from csv
df = pd.read_csv("data.csv", usecols=[2, 3])
# printing the dataframe
print(df)
Output:
Mentor price$
0 Robert 199
1 Elizibeth 299
2 Nolan 99
3 Chris 250
4 johnson 399