How to Fix Key Error in Pandas
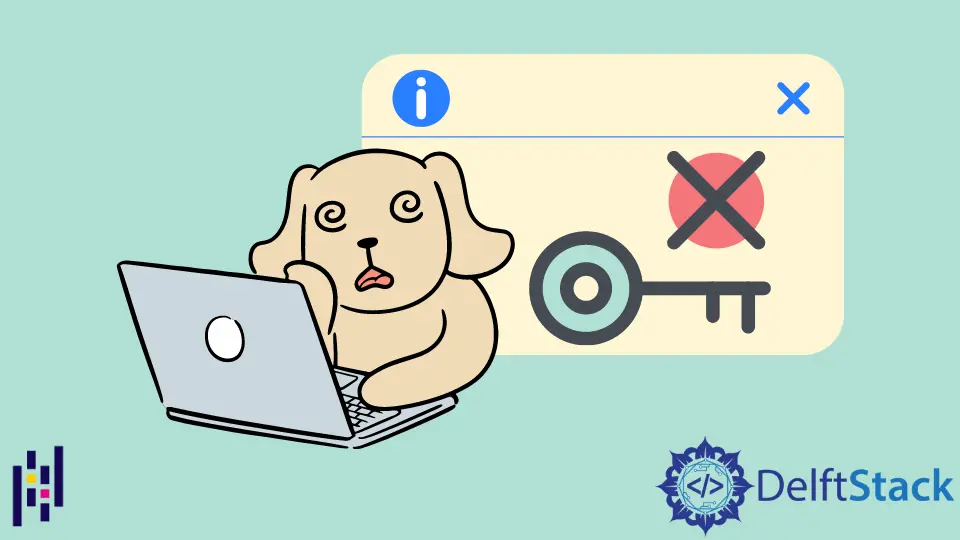
This tutorial explores the concept of a key error in Pandas.
What is the Key Error in Pandas
While working with Pandas, an analyst might encounter multiple errors that the code interpreter throws. These errors are widely ranged and would help us better investigate the issue.
In this tutorial, we aim to better understand the key error thrown by Pandas, the reason behind it throwing that error and the potential ways by which this error can be resolved.
Firstly, let us understand what this error means. A key error means that the key or element you’re trying to look for in the data frame or maybe even a column of a data frame does not exist.
Meaning you’re trying to query through or look for something in something that does not exist in the way you expect it to. Under such a situation, we have to face the key error in Pandas.
Analysts commonly face this error; it’s omnipresent in poorly formatted or labeled data frames. Now, let us understand why this error comes into the picture.
However, before we do that, let us create a dummy data frame to work with. We will call this data frame dat1
.
Let us create this data frame using the following code.
import pandas as pd
dat1 = pd.DataFrame({"dat1": [9, 5]})
The above code creates a data frame and a few entries, namely 9
and 5
. To view the entries in the data, we use the following code.
print(dat1)
The above code gives the following output.
dat1
0 9
1 5
As shown, we have 2 columns and 2 rows where one indicates the index and the second indicates the values in our data frame.
Why Do We Get a Key Error in Pandas
Now let us replicate the error. We can do this using the following code.
print(dat1["Date"])
The code tries to fetch the column named Date
from the dat1
data frame, which in theory does not exist. Thus we get the following output.
KeyError: 'Date'
Traceback (most recent call last)
Thus, we can see that if we access a data frame via a column name that does not exist, we might have to face the key error.
This error might appear because you might not have the entire data frame you’re trying to reference. Under such a situation, a key error is thrown that makes it difficult for the analyst to understand the exact reason behind it.
It’s best to check all the variable names before referencing or querying through data that does not exist in theory to avoid this scenario.
Thus, this tutorial has taught us the meaning, cause and potential solution regarding the key error thrown in Pandas.