How to Apply the If-Else Condition in a Pandas DataFrame
-
Use
DataFrame.loc[]
to Apply theif-else
Condition in a Pandas DataFrame in Python -
Use
DataFrame.apply()
to Apply theif-else
Condition in a Pandas DataFrame in Python -
Use
NumPy.select()
to Apply theif-else
Condition in a Pandas DataFrame in Python -
Use
lambda
Withapply()
to Apply theif-else
Condition in a Pandas DataFrame in Python
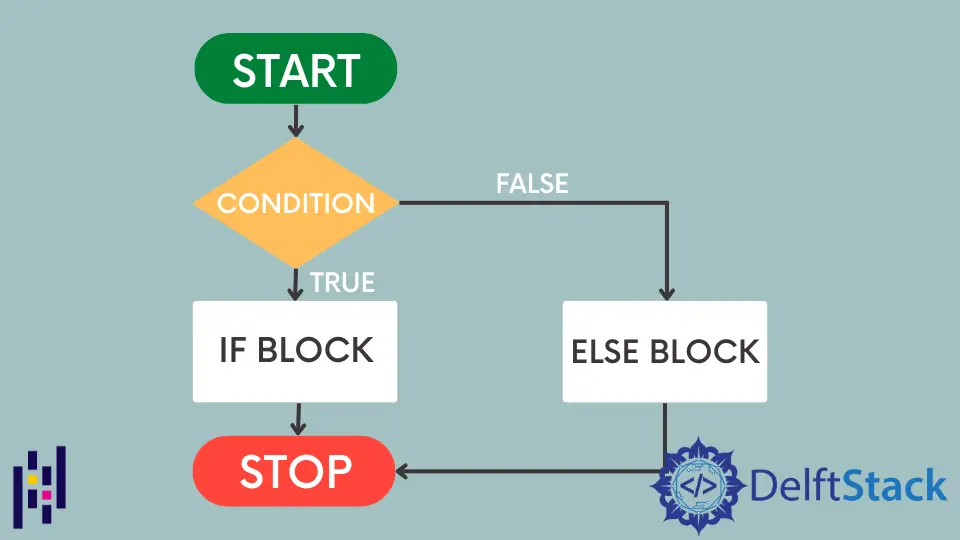
Pandas is an open-source data analysis library in Python. It provides many built-in methods to perform operations on numerical data.
In some cases, we want to apply the if-else
conditions on a Pandas dataframe to filter the records or perform computations according to some conditions. Python provides many ways to use if-else
on a Pandas dataframe.
Use DataFrame.loc[]
to Apply the if-else
Condition in a Pandas DataFrame in Python
loc[]
is a property of the Pandas data frame used to select or filter a group of rows or columns. In the following example, we will employ this property to filter the records that meet a given condition.
Here, we have a Pandas data frame consisting of the students’ data. Using loc[]
, we can only apply a single condition at a time.
We will filter those students having marks greater than or equal to 60 in the first condition and assign their result as Pass
in the new column Result
. Similarly, we will set Fail
for the rest of the student’s results in another condition.
Example Code:
# Python 3.x
import pandas as pd
df = pd.DataFrame(
{"Name": ["Robert", "Sam", "Alia", "Jhon", "Smith"], "Marks": [60, 41, 79, 51, 88]}
)
display(df)
df.loc[df["Marks"] >= 60, "Result"] = "Pass"
df.loc[df["Marks"] < 60, "Result"] = "Fail"
display(df)
Output:
Use DataFrame.apply()
to Apply the if-else
Condition in a Pandas DataFrame in Python
The apply()
method uses the data frame’s axis (row or column) to apply a function. We can make our defined function that consists of if-else
conditions and apply it to the Pandas dataframe.
Here, we have defined a function assign_Result()
and applied it to the Marks
column. The function consists of if-else
conditions that assign the result based on the Marks
and invoke this for every column row.
Example Code:
# Python 3.x
import pandas as pd
df = pd.DataFrame(
{"Name": ["Robert", "Sam", "Alia", "Jhon", "Smith"], "Marks": [60, 41, 79, 51, 88]}
)
display(df)
def assign_Result(marks):
if marks >= 60:
result = "Pass"
else:
result = "Fail"
return result
df["Result"] = df["Marks"].apply(assign_Result)
display(df)
Output:
Use NumPy.select()
to Apply the if-else
Condition in a Pandas DataFrame in Python
We can define multiple conditions for a column in a list and their corresponding values in another list if the condition is True
. The select()
method takes the list of conditions and their corresponding list of values as arguments and assigns them to the Result
column.
Example Code:
# Python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(
{"Name": ["Robert", "Sam", "Alia", "Jhon", "Smith"], "Marks": [60, 41, 79, 51, 88]}
)
display(df)
conditions = [(df["Marks"] >= 60), (df["Marks"] < 60)]
result = ["Pass", "Fail"]
df["Result"] = np.select(conditions, result)
display(df)
Output:
Use lambda
With apply()
to Apply the if-else
Condition in a Pandas DataFrame in Python
A lambda
is a small anonymous function consisting of a single expression. We will use lambda
with apply()
on the Marks
column.
The x
contains the marks in the lambda
expression. We applied the if-else
condition to the x
and assigned the result accordingly in the Result
column.
Example Code:
# Python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(
{"Name": ["Robert", "Sam", "Alia", "Jhon", "Smith"], "Marks": [60, 41, 79, 51, 88]}
)
display(df)
df["Result"] = df["Marks"].apply(lambda x: "Pass" if x >= 60 else "Fail")
display(df)
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn