How to Exclude Pandas DataFrame Column
-
Pandas Select All Except One Column Using the
loc
Property -
Pandas Select All Except One Column Using the
drop()
Method -
Pandas Select All Except One Column Using the
difference()
Method
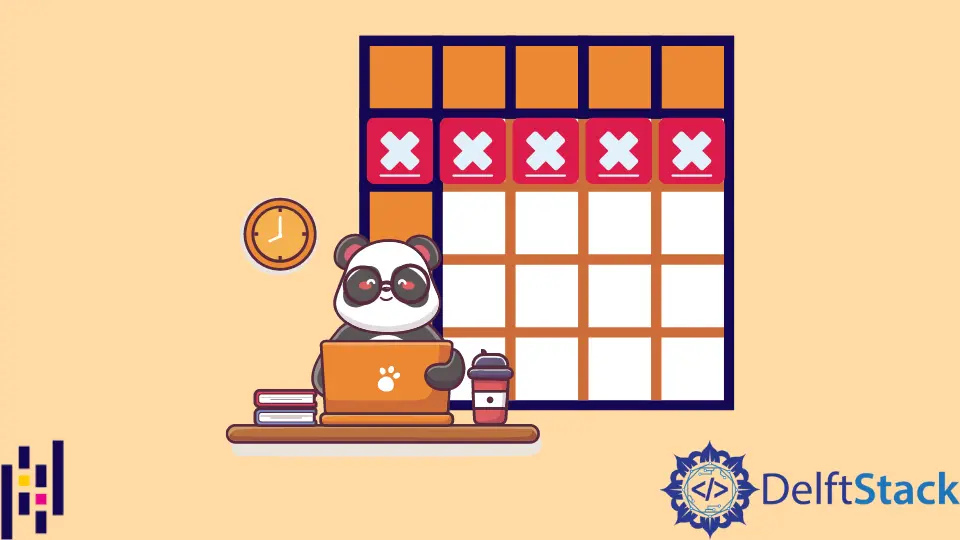
This tutorial explains how we can select all columns except one from a particular DataFrame. We will use the below example DataFrame in this article.
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Amazon", "Tesla", "Facebook", "Boeing"],
"Price(in $)": [3180, 835, 267, 209],
"Sector": ["Technology", "Technology", "Technology", "Aircraft"],
}
)
print("Stocks Dataframe:")
print(stocks_df, "\n")
Output:
Stocks Dataframe:
Stock Price(in $) Sector
0 Amazon 3180 Technology
1 Tesla 835 Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
Pandas Select All Except One Column Using the loc
Property
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Amazon", "Tesla", "Facebook", "Boeing"],
"Price(in $)": [3180, 835, 267, 209],
"Sector": ["Technology", "Technology", "Technology", "Aircraft"],
}
)
print("Stocks Dataframe:")
print(stocks_df, "\n")
print("Stocks DataFrame excluding Sector Column:")
filtered_df = stocks_df.loc[:, stocks_df.columns != "Sector"]
print(filtered_df, "\n")
Output:
Stocks Dataframe:
Stock Price(in $) Sector
0 Amazon 3180 Technology
1 Tesla 835 Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
Stocks DataFrame excluding Sector Column:
Stock Price(in $)
0 Amazon 3180
1 Tesla 835
2 Facebook 267
3 Boeing 209
It selects all except the Sector
column from the stocks_df
DataFrame, assigns the result to filtered_df
, and then displays the content of the filetered_df
.
The loc
property selects the elements based on specified rows and columns. The :
symbol before ,
in loc
property specifies we need to select all the rows. For the columns, we have specified to select only the column whose name is not Sector
. Hence, it will select all the columns except the Sector
column.
Pandas Select All Except One Column Using the drop()
Method
We can drop specified columns from a DataFrame using the drop()
method by setting axis=1
in the method.
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Amazon", "Tesla", "Facebook", "Boeing"],
"Price(in $)": [3180, 835, 267, 209],
"Sector": ["Technology", "Technology", "Technology", "Aircraft"],
}
)
print("Stocks Dataframe:")
print(stocks_df, "\n")
print("Stocks DataFrame excluding Sector Column:")
filtered_df = stocks_df.drop("Sector", axis=1)
print(filtered_df, "\n")
Output:
Stocks Dataframe:
Stock Price(in $) Sector
0 Amazon 3180 Technology
1 Tesla 835 Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
Stocks DataFrame excluding Sector Column:
Stock Price(in $)
0 Amazon 3180
1 Tesla 835
2 Facebook 267
3 Boeing 209
It drops the Sector
column from the stocks_df
DataFrame and assigns the result to filtered_df
.
We can also exclude multiple columns from a DataFrame by dropping multiple columns using the drop()
method. We provide a list of columns’ names to be dropped as an argument to the drop()
method.
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Amazon", "Tesla", "Facebook", "Boeing"],
"Price(in $)": [3180, 835, 267, 209],
"Sector": ["Technology", "Technology", "Technology", "Aircraft"],
}
)
print("Stocks Dataframe:")
print(stocks_df, "\n")
print("Stocks DataFrame excluding Sector and Price Column:")
filtered_df = stocks_df.drop(["Sector", "Price(in $)"], axis=1)
print(filtered_df, "\n")
Output:
Stocks Dataframe:
Stock Price(in $) Sector
0 Amazon 3180 Technology
1 Tesla 835 Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
Stocks DataFrame excluding Sector and Price Column:
Stock
0 Amazon
1 Tesla
2 Facebook
3 Boeing
It excludes the columns Price(in $)
and Sector
from the stocks_df
DataFrame.
Pandas Select All Except One Column Using the difference()
Method
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Amazon", "Tesla", "Facebook", "Boeing"],
"Price(in $)": [3180, 835, 267, 209],
"Sector": ["Technology", "Technology", "Technology", "Aircraft"],
}
)
print("Stocks Dataframe:")
print(stocks_df, "\n")
print("Stocks DataFrame excluding Sector Column:")
filtered_df = stocks_df[stocks_df.columns.difference(["Sector"])]
print(filtered_df, "\n")
Output:
Stocks Dataframe:
Stock Price(in $) Sector
0 Amazon 3180 Technology
1 Tesla 835 Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
Stocks DataFrame excluding Sector Column:
Price(in $) Stock
0 3180 Amazon
1 835 Tesla
2 267 Facebook
3 209 Boeing
It drops the Sector
column from the stocks_df
DataFrame and assigns the result to filtered_df
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInRelated Article - Pandas DataFrame Column
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Get the Sum of Pandas Column
- How to Change the Order of Pandas DataFrame Columns
- How to Convert DataFrame Column to String in Pandas