How to Calculate Exponential Moving Average Values in Pandas
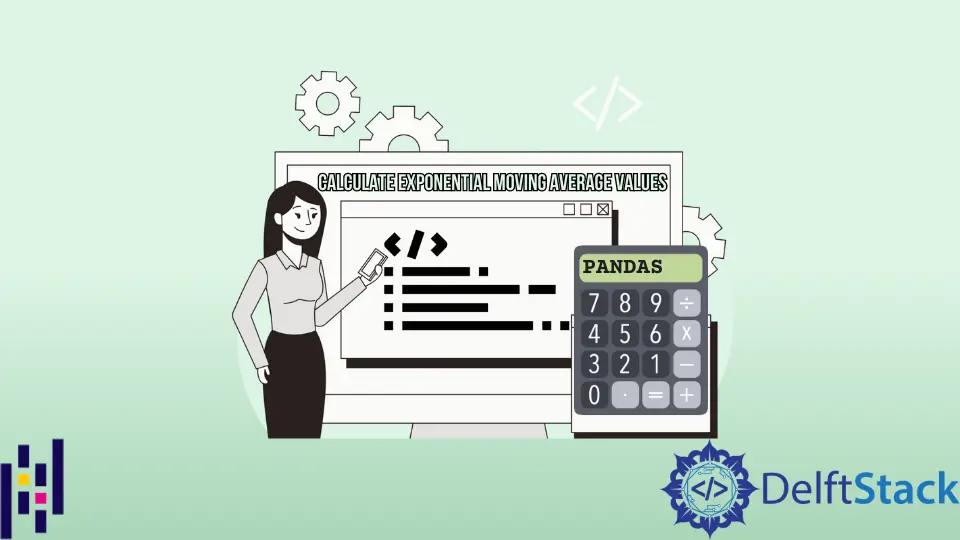
This tutorial will discuss calculating the ewm (exponential moving average) in Pandas.
Steps to Calculate Exponential Moving Average Values in Pandas
The following are the steps to find ewm values in Pandas.
Import Pandas
We will need to import pandas to get started.
import pandas as pd
Create Pandas DataFrame
Let us now create a sample dataframe with column prices to calculate ewm.
data = {"prices": [22.27, 22.19, 22.08, 22.17, 22.18]}
df = pd.DataFrame(data)
Let us take a look at our dataframe.
print(df)
Output:
prices
0 22.27
1 22.19
2 22.08
3 22.17
4 22.18
Use the rolling()
Function to Divide DataFrame
We will now use the rolling()
function to roll our dataframe with a defined span size and divide our dataframe into two dataframes.
span = 2
sma = df.rolling(window=span, min_periods=span).mean()[:span]
We pass the window size and min_periods
parameters to the rolling()
function, a predefined variable.
Let us now insert the rest of our dataframe into a separate dataframe.
rest = df[span:]
Use the pd.concat()
and ewm()
Functions to Calculate the Exponential Moving Average
Now that we have successfully divided our default dataframe, we will use the pd.concat()
and ewm()
functions to calculate the exponential moving average in our dataframe column.
ewm1 = pd.concat([sma, rest]).ewm(span=span, adjust=False).mean()
We calculated ewm using the ewm()
function in the above code.
We passed the span
parameter. Also, the adjust
parameter is passed as False to prevent accounting for imbalance in relative weightings in beginning periods.
Let us now print the ewm values to see the output.
print(ewm1)
Output:
prices
0 NaN
1 22.230000
2 22.130000
3 22.156667
4 22.172222
As seen in the above output, we have successfully calculated the ewm values for the sample dataframe. Thus, we can successfully find the ewm values in a Pandas dataframe.