How to Convert a Pandas Series of Datetime to String in Python
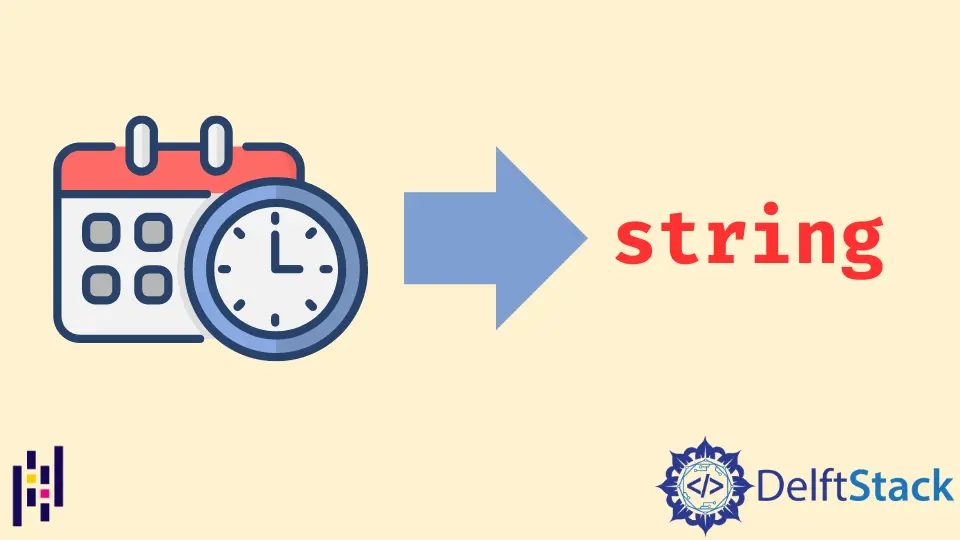
Pandas Series is a one-dimensional array that can hold any data type along with labels. Suppose you have a pandas series of datetime
objects. We can convert a datatime
object to its string equivalent using the strftime()
function and some format codes. But to convert the datetime
objects of a pandas series, the approach to be followed is a bit different. This article will talk about how we can carry out such a conversion.
Convert a Pandas Series of datetime
Objects to Their String Equivalents
Refer to the following code. It first creates a pandas
Series of datetime
objects and then converts them to a pandas
series of string objects.
import pandas as pd
dates = pd.to_datetime(
pd.Series(["01/01/2021", "02/02/2021", "03/03/2021", "04/04/2021", "05/05/2021"]),
format="%d/%m/%Y",
)
print("Before conversion")
print(dates)
print("After conversion")
dates = dates.dt.strftime("%Y-%m-%d")
print(dates)
Output:
Before conversion
0 2021-01-01
1 2021-02-02
2 2021-03-03
3 2021-04-04
4 2021-05-05
dtype: datetime64[ns]
After conversion
0 2021-01-01
1 2021-02-02
2 2021-03-03
3 2021-04-04
4 2021-05-05
dtype: object
Note the dtype
value of output. The former one shows that the series is of datetime
objects, and the latter indicates that the series is of string
objects.
We can also use the lambda
function to convert the data type of the objects. Refer to the following code for the same. The lambda
function utilizes the strftime()
function to perform the conversion.
import pandas as pd
dates = pd.to_datetime(
pd.Series(["01/01/2021", "02/02/2021", "03/03/2021", "04/04/2021", "05/05/2021"]),
format="%d/%m/%Y",
)
print("Before conversion")
print(dates)
print("After conversion")
dates = dates.apply(lambda x: x.strftime("%Y-%m-%d"))
print(dates)
Output:
Before conversion
0 2021-01-01
1 2021-02-02
2 2021-03-03
3 2021-04-04
4 2021-05-05
dtype: datetime64[ns]
After conversion
0 2021-01-01
1 2021-02-02
2 2021-03-03
3 2021-04-04
4 2021-05-05
dtype: object