How to Calculate Cross Join Between Two DataFrames in Pandas
-
Method 1: Using the
merge
Function -
Method 2: Using the
assign
andmerge
Combination -
Method 3: Using
concat
withrepeat
- Conclusion
- FAQ
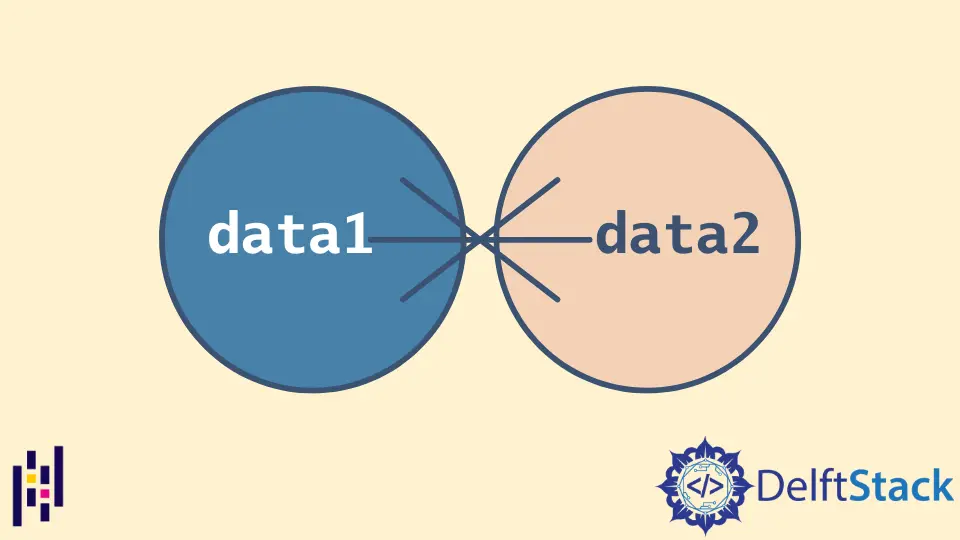
In the world of data analysis, merging datasets can often be a crucial step in deriving meaningful insights. One such method is the cross join, which allows you to combine every row from one DataFrame with every row from another.
This tutorial will guide you through the steps to calculate a cross join between two DataFrames using Pandas, a powerful data manipulation library in Python. Whether you’re dealing with sales data, customer information, or product listings, understanding how to perform a cross join can significantly enhance your data analysis capabilities. Let’s dive in and explore the various methods to achieve this in Pandas.
Method 1: Using the merge
Function
The merge
function in Pandas is a versatile tool that allows you to join DataFrames in various ways, including a cross join. To perform a cross join, you can set the how
parameter to 'cross'
. This method is straightforward and efficient for combining two DataFrames.
Here’s how you can do it:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']
})
cross_join = pd.merge(df1, df2, how='cross')
print(cross_join)
Output:
A B C D
0 A0 B0 C0 D0
1 A0 B0 C1 D1
2 A1 B1 C0 D0
3 A1 B1 C1 D1
4 A2 B2 C0 D0
5 A2 B2 C1 D1
In this example, we created two DataFrames, df1
and df2
. The merge
function is then called with the how='cross'
parameter, which results in a DataFrame where every combination of rows from df1
and df2
is present. This method is not only easy to implement but also very readable, making it an excellent choice for those new to Pandas.
Method 2: Using the assign
and merge
Combination
Another effective way to achieve a cross join is to use the assign
method in conjunction with merge
. This approach involves adding a temporary column to both DataFrames, which helps in performing the cross join.
Here’s how you can implement this method:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']
})
df1['key'] = 1
df2['key'] = 1
cross_join = pd.merge(df1, df2, on='key').drop('key', axis=1)
print(cross_join)
Output:
A B C D
0 A0 B0 C0 D0
1 A0 B0 C1 D1
2 A1 B1 C0 D0
3 A1 B1 C1 D1
4 A2 B2 C0 D0
5 A2 B2 C1 D1
In this method, we first add a temporary column named key
with a constant value of 1 to both DataFrames. This allows us to merge them on this common key, effectively creating a cross join. After the merge, we drop the temporary key column to tidy up the resulting DataFrame. This method is particularly useful when you want to maintain control over the merging process and require more flexibility.
Method 3: Using concat
with repeat
If you prefer a more manual approach, you can achieve a cross join using the concat
function along with repeat
. This method is slightly more complex but provides a clear understanding of how cross joins work under the hood.
Here’s how to do it:
import pandas as pd
df1 = pd.DataFrame({
'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']
})
df2 = pd.DataFrame({
'C': ['C0', 'C1'],
'D': ['D0', 'D1']
})
cross_join = pd.concat([df1.loc[df1.index.repeat(len(df2))].reset_index(drop=True),
df2.loc[df2.index.repeat(len(df1))].reset_index(drop=True)], axis=1)
print(cross_join)
Output:
A B C D
0 A0 B0 C0 D0
1 A0 B0 C1 D1
2 A1 B1 C0 D0
3 A1 B1 C1 D1
4 A2 B2 C0 D0
5 A2 B2 C1 D1
In this approach, we repeat the indices of both DataFrames based on the length of the other DataFrame. By using concat
, we can then combine them side by side. This method can be more intuitive for those who are comfortable with manipulating indices and may offer more control over how the DataFrames are combined.
Conclusion
In summary, calculating a cross join between two DataFrames in Pandas can be achieved through various methods, each with its own advantages. Whether you choose to use the merge
function, the combination of assign
and merge
, or the concat
method with repeated indices, understanding these techniques will help you effectively manage and analyze your datasets. With the ability to create comprehensive combinations of data, you can unlock new insights and enhance your data analysis projects.
FAQ
-
What is a cross join in Pandas?
A cross join in Pandas combines every row from one DataFrame with every row from another, resulting in a Cartesian product. -
Can I perform a cross join without using the
merge
function?
Yes, you can use methods likeconcat
with repeated indices or add a temporary key column to achieve a cross join. -
Is a cross join always necessary for data analysis?
Not always. Cross joins can lead to large datasets, so they should be used judiciously based on the analysis requirements. -
How does a cross join differ from an inner join?
A cross join combines all rows, while an inner join only combines rows that meet a specified condition. -
Can I apply filters after performing a cross join?
Yes, you can apply filters to the resulting DataFrame after the cross join to focus on specific data points.